python不同时间戳的折线画在一张图上
时间: 2023-06-12 10:02:10 浏览: 53
您好,感谢您的提问。您可以使用Matplotlib库来实现将不同时间戳的折线画在一张图上。
首先,您需要将不同时间戳对应的数据存储在列表中,例如:
```
x1 = [1, 2, 3, 4, 5]
y1 = [10, 8, 6, 4, 2]
x2 = [1, 2, 3, 4, 5]
y2 = [2, 4, 6, 8, 10]
```
然后,您可以使用Matplotlib的plot()函数来绘制折线图,如下所示:
```
import matplotlib.pyplot as plt
plt.plot(x1, y1, label='Line 1')
plt.plot(x2, y2, label='Line 2')
plt.xlabel('Time')
plt.ylabel('Value')
plt.title('Multiple Lines on One Graph')
plt.legend()
plt.show()
```
其中,plot()函数的第一个参数是x轴数据,第二个参数是y轴数据,label参数用于设置线条的标签,xlabel()和ylabel()函数用于设置坐标轴标签,title()函数用于设置图表标题,legend()函数用于显示线条标签。
希望这个回答可以帮到您,如果您有任何疑问,请随时追问。
相关问题
python横坐标为时间戳 间隔不同的折线画在一张图上
您可以使用Matplotlib库来实现这个任务。以下是基本的代码示例:
```python
import matplotlib.pyplot as plt
import numpy as np
# 创建时间戳数组和对应的y值数组
x1 = [1, 2, 3, 4, 5, 6]
y1 = [10, 20, 30, 40, 50, 60]
x2 = [1, 3, 5, 7, 9]
y2 = [15, 25, 35, 45, 55]
# 绘制折线图
plt.plot(x1, y1, label='Line 1')
plt.plot(x2, y2, label='Line 2')
# 添加标题、轴标签和图例
plt.title('Multiple lines plot')
plt.xlabel('Time')
plt.ylabel('Value')
plt.legend()
# 显示图形
plt.show()
```
在这个示例中,我们创建了两个时间戳数组x1和x2和对应的y值数组y1和y2。然后,我们绘制这两条折线,并添加标题、轴标签和图例。最后,我们使用plt.show()命令显示图形。
如果您需要使用不同的间隔来绘制折线图,可以考虑对x轴上的值进行修改。例如,您可以使用np.linspace()函数来创建等间隔的时间戳。例如:
```python
# 创建等间隔的时间戳数组
x1 = np.linspace(0, 10, 6)
y1 = [10, 20, 30, 40, 50, 60]
x2 = np.linspace(0, 10, 5)
y2 = [15, 25, 35, 45, 55]
```
在这个示例中,我们使用np.linspace()函数来创建在0和10之间6个等间隔的时间戳,以及在0和10之间5个等间隔的时间戳。然后,我们使用这些时间戳和对应的y值来绘制折线图。
python横坐标为时间戳画多个折线图
好的,理解了你的要求。针对你的问题,首先要确保你的数据是按时间顺序排列的。然后可以使用Python的Matplotlib库来绘制折线图,以下是示例代码:
```Python
import matplotlib.pyplot as plt
# 时间戳列表
timestamps = [1609459200, 1609545600, 1609632000, 1609718400, 1609804800]
# 折线图数据,可以有多组数据
line1 = [10, 12, 8, 15, 11]
line2 = [5, 8, 6, 9, 10]
line3 = [17, 13, 16, 11, 14]
# 通过时间戳转换成可读的时间格式
ticks = []
for ts in timestamps:
ticks.append(time.strftime("%Y-%m-%d", time.localtime(ts)))
# 绘制折线图
plt.plot(ticks, line1, label='Line1')
plt.plot(ticks, line2, label='Line2')
plt.plot(ticks, line3, label='Line3')
# 调整x轴的坐标文字角度
plt.xticks(rotation=45)
# 添加图例,展示每条折线的含义
plt.legend()
# 设置图表标题和坐标轴标签
plt.title('Multiple Line Chart with Timestamp on X-Axis')
plt.xlabel('Time')
plt.ylabel('Value')
# 显示图表
plt.show()
```
运行以上代码即可在Python中绘制多个折线图,并在x轴上显示时间戳。希望我的回答对你有所帮助。
相关推荐
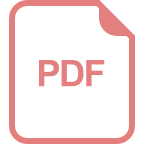












