生成一个以图搜图的python代码
时间: 2024-03-11 12:37:02 浏览: 29
以下是一个使用百度AI开放平台的图像搜索API实现以图搜图的Python代码示例:
```python
import requests
import base64
# 百度AI开放平台图像搜索API接口地址
url = "https://aip.baidubce.com/rest/2.0/image-classify/v1/realtime_search/similar/search"
# 百度AI开放平台API Key和Secret Key
api_key = "your_api_key"
secret_key = "your_secret_key"
# 读取待搜索的图片文件
with open("image.jpg", "rb") as f:
image_data = f.read()
# 将图片数据转换为base64编码格式
image_base64 = base64.b64encode(image_data).decode()
# 构造API请求的参数
params = {
"image": image_base64,
"quality_control": "HIGH",
"detect_direction": "true"
}
# 使用API Key和Secret Key进行身份验证,获取Access Token
auth_url = "https://aip.baidubce.com/oauth/2.0/token"
auth_params = {
"grant_type": "client_credentials",
"client_id": api_key,
"client_secret": secret_key
}
auth_response = requests.post(auth_url, data=auth_params)
access_token = auth_response.json()["access_token"]
# 发送API请求,获取搜索结果
headers = {"Content-Type": "application/x-www-form-urlencoded"}
params["access_token"] = access_token
response = requests.post(url, headers=headers, data=params)
result = response.json()
# 输出搜索结果
print(result)
```
在代码中,需要替换以下部分:
- `your_api_key`和`your_secret_key`为在百度AI开放平台注册并创建的应用所对应的API Key和Secret Key。
- `"image.jpg"`为待搜索的图片文件路径,可以根据实际情况进行修改。
注意:使用百度AI开放平台的API需要进行身份验证,因此需要在代码中调用Access Token接口获取Access Token,并将Access Token作为请求参数发送给图像搜索API。Access Token的有效期为30天,过期后需要重新获取。
相关推荐
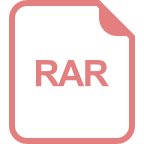
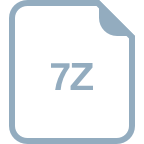














