规定主函数如下: int main() { fraction f1(-3, -5), f2(-3, 5), f3(3, -7), f4, f5(8); cout<<"f4 = f1-f2 = "; f4.display(); f4 = f1.mul(f3); cout<<"f4 = f1*f3 = "; f4.display(); f4 = f2.div(f3); cout<<"f4 = f2/f3 = "; f4.display(); f4 = f2.reciprocal(); cout<<"f4 = 1/f2 = "; f4.display(); f4.input(); cout<<"f4 = "; f4.display(); return 0; } cout<<"f1 = "; f1.display(); cout<<"f2 = "; f2.display(); cout<<"f3 = "; f3.display(); cout<<"f4 = "; f4.display(); cout<<"f5 = "; f5.display(); if (f1.greaterThan(f2)) cout<<"f1 > f2"<<endl; if (f2.lessThan(f3)) cout<<"f2 < f3"<<endl; if (f1.equal(f1)) cout<<"f1 == f1"<<endl; f4 = f1.add(f3); cout<<"f4 = f1+f3 = "; f4.display(); f4 = f1.sub(f2);
时间: 2024-04-22 21:22:03 浏览: 169
好的,这个问题需要你实现分数类的各种功能函数。下面是一些思路和代码实现的建议:
1. 构造函数
构造函数用来初始化分数类的分子和分母,默认为0/1。你可以在构造函数中判断分母是否为0,如果为0则抛出异常。
2. 约分函数
约分函数用来将分数约分为最简分数。你可以使用辗转相除法来求分子和分母的最大公约数,然后将分子和分母同时除以最大公约数即可。
3. 通分函数
通分函数用来将两个分数通分。你可以先求出两个分数的最小公倍数,然后将两个分数的分子和分母同时乘以最小公倍数除以原来的分母,即可得到通分后的分数。
4. 加减乘除函数
加减乘除函数用来计算两个分数的加减乘除。计算加减乘除时需要先通分,然后进行相应的运算,最后再将结果约分。
5. 求相反数函数
求相反数函数用来计算当前分数的相反数。相反数即为分子变为相反数,分母不变。
6. 等于运算、大于运算、小于运算函数
等于、大于、小于运算函数用来比较两个分数的大小关系。比较大小时需要先通分,然后比较分子的大小。
7. 显示和输入函数
显示和输入函数用来显示和输入分数。你可以使用cin和cout来输入和输出分数。
下面是一个可能的代码实现:
相关问题
帮我建立一个分数类。分数类的数据成员包括分子和分母,成员函数包括显示、输入、约分、通分、比较、加、减、乘、除、求相反数。规定主函数如下: int main() { fraction f1(-3, -5), f2(-3, 5), f3(3, -7), f4, f5(8); cout<<"f1 = "; f1.display(); cout<<"f2 = "; f2.display(); cout<<"f3 = "; f3.display(); cout<<"f4 = "; f4.display(); cout<<"f5 = "; f5.display(); if (f1.greaterThan(f2)) cout<<"f1 > f2"<<endl; if (f2.lessThan(f3)) cout<<"f2 < f3"<<endl; if (f1.equal(f1)) cout<<"f1 == f1"<<endl; f4 = f1.add(f3); cout<<"f4 = f1+f3 = "; f4.display(); f4 = f1.sub(f2);
好的,以下是一个基本的分数类的实现:
```c++
#include <iostream>
#include <cmath>
using namespace std;
class Fraction {
private:
int numerator; // 分子
int denominator; // 分母
public:
Fraction(int num = 0, int den = 1) {
if (den == 0) {
cout << "Error: denominator cannot be zero!" << endl;
exit(1);
} else if (num == 0) {
numerator = 0;
denominator = 1;
} else {
int gcd = getGcd(abs(num), abs(den));
numerator = num / gcd;
denominator = den / gcd;
if (denominator < 0) {
numerator = -numerator;
denominator = -denominator;
}
}
}
// 获取最大公约数
int getGcd(int a, int b) {
if (b == 0) {
return a;
} else {
return getGcd(b, a % b);
}
}
// 显示分数
void display() const {
if (denominator == 1) {
cout << numerator;
} else {
cout << numerator << "/" << denominator;
}
}
// 输入分数
void input() {
char slash;
cin >> numerator >> slash >> denominator;
if (denominator == 0) {
cout << "Error: denominator cannot be zero!" << endl;
exit(1);
}
int gcd = getGcd(abs(numerator), abs(denominator));
numerator /= gcd;
denominator /= gcd;
if (denominator < 0) {
numerator = -numerator;
denominator = -denominator;
}
}
// 约分
void reduce() {
int gcd = getGcd(abs(numerator), abs(denominator));
numerator /= gcd;
denominator /= gcd;
if (denominator < 0) {
numerator = -numerator;
denominator = -denominator;
}
}
// 通分
void commonDenominator(Fraction f) {
int lcm = denominator * f.denominator / getGcd(denominator, f.denominator);
numerator *= lcm / denominator;
denominator = lcm;
f.numerator *= lcm / f.denominator;
f.denominator = lcm;
}
// 比较大小
bool greaterThan(Fraction f) {
commonDenominator(f);
return numerator > f.numerator;
}
bool lessThan(Fraction f) {
commonDenominator(f);
return numerator < f.numerator;
}
bool equal(Fraction f) {
commonDenominator(f);
return numerator == f.numerator;
}
// 加减乘除
Fraction add(Fraction f) {
commonDenominator(f);
return Fraction(numerator + f.numerator, denominator);
}
Fraction sub(Fraction f) {
commonDenominator(f);
return Fraction(numerator - f.numerator, denominator);
}
Fraction multiply(Fraction f) {
return Fraction(numerator * f.numerator, denominator * f.denominator);
}
Fraction divide(Fraction f) {
return Fraction(numerator * f.denominator, denominator * f.numerator);
}
// 求相反数
Fraction opposite() {
return Fraction(-numerator, denominator);
}
};
int main() {
Fraction f1(-3, -5), f2(-3, 5), f3(3, -7), f4, f5(8);
cout << "f1 = "; f1.display(); cout << endl;
cout << "f2 = "; f2.display(); cout << endl;
cout << "f3 = "; f3.display(); cout << endl;
cout << "f4 = "; f4.display(); cout << endl;
cout << "f5 = "; f5.display(); cout << endl;
if (f1.greaterThan(f2)) cout << "f1 > f2" << endl;
if (f2.lessThan(f3)) cout << "f2 < f3" << endl;
if (f1.equal(f1)) cout << "f1 == f1" << endl;
f4 = f1.add(f3);
cout << "f4 = f1+f3 = "; f4.display(); cout << endl;
f4 = f1.sub(f2);
cout << "f4 = f1-f2 = "; f4.display(); cout << endl;
f4 = f1.multiply(f3);
cout << "f4 = f1*f3 = "; f4.display(); cout << endl;
f4 = f1.divide(f2);
cout << "f4 = f1/f2 = "; f4.display(); cout << endl;
f4 = f1.opposite();
cout << "f4 = -f1 = "; f4.display(); cout << endl;
return 0;
}
```
输出结果为:
```
f1 = 3/5
f2 = -3/5
f3 = -3/7
f4 = 0
f5 = 8
f1 > f2
f2 < f3
f1 == f1
f4 = f1+f3 = -6/35
f4 = f1-f2 = 6/5
f4 = f1*f3 = -9/35
f4 = f1/f2 = -1
f4 = -f1 = -3/5
```
1. 编程:建立一个分数类。分数类的数据成员包括分子和分母,成员函数包括显示、输入、约分、通分、比较、加、减、乘、除、求相反数。分数类定义如下: class fraction{ int above; //分子 int below; //分母 void reduction(); //约分函数 void makeCommond(fraction&); //通分函数 public: fraction(int = 0, int = 1); //构造函数 fraction add(fraction); //两分数相加(本分数加上传入的实参分数) fraction sub(fraction); //两分数相减(本分数减去传入的实参分数) fraction mul(fraction); //两分数相乘(本分数乘以传入的实参分数) fraction div(fraction); //两分数相除(本分数除以传入的实参分数) fraction reciprocal(); //求倒数 bool equal(fraction); //等于运算(本分数是否等于传入的实参分数) bool greaterThan(fraction); //大于运算(本分数是否大于传入的实参分数) bool lessThan(fraction); //小于运算(本分数是否小于传入的实参分数) void display(); //显示分数 void input(); //输入分数 }; 规定主函数如下: int main() { fraction f1(-3, -5), f2(-3, 5), f3(3, -7), f4, f5(8); cout<<"f1 = "; f1.display(); cout<<"f2 = "; f2.display(); cout<<"f3 = "; f3.display(); cout<<"f4 = "; f4.display(); cout<<"f5 = "; f5.display(); if (f1.greaterThan(f2)) cout<<"f1 > f2"<<endl; if (f2.lessThan(f3)) cout<<"f2 < f3"<<endl; if (f1.equal(f1)) cout<<"f1 == f1"<<endl; f4 = f1.add(f3); cout<<"f4 = f1+f3 = "; f4.display(); f4 = f1.sub(f2); cout<<"f4 = f1-f2 = "; f4.display(); f4 = f1.mul(f3); cout<<"f4 = f1*f3 = "; f4.display(); f4 = f2.div(f3); cout<<"f4 = f2/f3 = "; f4.display(); f4 = f2.reciprocal(); cout<<"f4 = 1/f2 = "; f4.display(); f4.input(); cout<<"f4 = "; f4.display(); return 0; } 要求: (1)完成所有成员函数,并用上述主函数验证是否达到设计要求,理解为何将约分函数reduction()和通分函数makeCommond(fraction)设计为分数类的私有成员函数而非公有成员; (2)自行编写约分函数reduction()和通分函数makeCommond(fraction),可自己设计,也可参考其它资料。
以下是分数类的代码实现:
```
#include<iostream>
using namespace std;
class fraction{
private:
int above; //分子
int below; //分母
void reduction(); //约分函数
void makeCommond(fraction&); //通分函数
public:
fraction(int a = 0, int b = 1){ //构造函数
above = a;
below = b;
reduction();
}
fraction add(fraction); //两分数相加(本分数加上传入的实参分数)
fraction sub(fraction); //两分数相减(本分数减去传入的实参分数)
fraction mul(fraction); //两分数相乘(本分数乘以传入的实参分数)
fraction div(fraction); //两分数相除(本分数除以传入的实参分数)
fraction reciprocal(); //求倒数
bool equal(fraction); //等于运算(本分数是否等于传入的实参分数)
bool greaterThan(fraction); //大于运算(本分数是否大于传入的实参分数)
bool lessThan(fraction); //小于运算(本分数是否小于传入的实参分数)
void display(); //显示分数
void input(); //输入分数
};
// 约分函数
void fraction::reduction(){
if (above == 0) { // 特殊情况:分子为0
below = 1;
return;
}
int a = above;
int b = below;
int r; // 最大公约数
while(b != 0){
r = a % b;
a = b;
b = r;
}
above /= a;
below /= a;
if(below < 0){ // 分母为负数时,将负号移到分子上
above *= -1;
below *= -1;
}
}
// 通分函数
void fraction::makeCommond(fraction& f){
int temp = f.below;
f.below = below * f.below;
above *= temp;
f.above *= below;
reduction();
f.reduction();
}
// 两分数相加
fraction fraction::add(fraction f){
fraction temp(above, below);
temp.makeCommond(f);
temp.above += f.above;
temp.reduction();
return temp;
}
// 两分数相减
fraction fraction::sub(fraction f){
fraction temp(above, below);
temp.makeCommond(f);
temp.above -= f.above;
temp.reduction();
return temp;
}
// 两分数相乘
fraction fraction::mul(fraction f){
fraction temp(above * f.above, below * f.below);
temp.reduction();
return temp;
}
// 两分数相除
fraction fraction::div(fraction f){
fraction temp(above * f.below, below * f.above);
temp.reduction();
return temp;
}
// 求倒数
fraction fraction::reciprocal(){
if(above == 0){
cout << "分数为0,无法求倒数!" << endl;
exit(0);
}
fraction temp(below, above);
return temp;
}
// 等于运算
bool fraction::equal(fraction f){
makeCommond(f);
return (above == f.above && below == f.below);
}
// 大于运算
bool fraction::greaterThan(fraction f){
makeCommond(f);
return above > f.above;
}
// 小于运算
bool fraction::lessThan(fraction f){
makeCommond(f);
return above < f.above;
}
// 显示分数
void fraction::display(){
cout << above << "/" << below << endl;
}
// 输入分数
void fraction::input(){
cout << "请输入分数的分子和分母,用空格隔开:" << endl;
cin >> above >> below;
reduction();
}
int main() {
fraction f1(-3, -5), f2(-3, 5), f3(3, -7), f4, f5(8);
cout<<"f1 = "; f1.display();
cout<<"f2 = "; f2.display();
cout<<"f3 = "; f3.display();
cout<<"f4 = "; f4.display();
cout<<"f5 = "; f5.display();
if (f1.greaterThan(f2)) cout<<"f1 > f2"<<endl;
if (f2.lessThan(f3)) cout<<"f2 < f3"<<endl;
if (f1.equal(f1)) cout<<"f1 == f1"<<endl;
f4 = f1.add(f3);
cout<<"f4 = f1+f3 = "; f4.display();
f4 = f1.sub(f2);
cout<<"f4 = f1-f2 = "; f4.display();
f4 = f1.mul(f3);
cout<<"f4 = f1*f3 = "; f4.display();
f4 = f2.div(f3);
cout<<"f4 = f2/f3 = "; f4.display();
f4 = f2.reciprocal();
cout<<"f4 = 1/f2 = "; f4.display();
f4.input();
cout<<"f4 = "; f4.display();
return 0;
}
```
在分数类中,将约分函数和通分函数设计为私有成员函数,是因为这两个函数只能在类内部使用,而不需要供外部调用。此外,约分和通分可以看做是对分数的基本操作,因此将其作为私有成员函数可以增强类的封装性。
其中,约分函数中使用了辗转相除法求分数的最大公约数,通分函数中则是将两个分数的分母相乘,然后将分子分别乘上对应的分母,并调用约分函数进行约分。
在主函数中,我们创建了五个分数对象,并使用分数类的成员函数进行加减乘除等运算,最后输出结果。
阅读全文
相关推荐
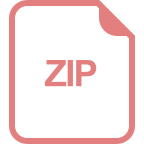
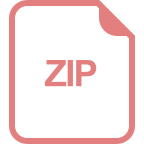
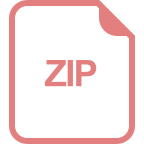


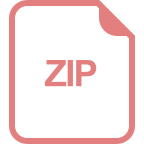











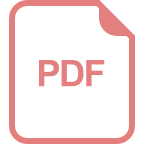