a = input() c = ['Math', 'Python Programming', 'Food science', 'Chinese'] for i in a: d=c[int(i)-1] print(d)修改代码报错
时间: 2024-06-09 13:11:46 浏览: 38
看起来代码中没有明显的语法错误,但是如果运行时报错的话,有可能是因为以下原因导致的:
1. input() 函数没有输入任何的参数,所以在运行程序时需要手动输入一个字符串。如果没有输入任何内容,a 变量将会是空字符串,而且在执行 for i in a: 语句时会产生错误。
2. 如果在手动输入参数时输入了一个非数字的字符,例如字母或符号,那么在执行 int(i)-1 的时候会产生 TypeError 错误。
3. 当输入的数值不在 c 列表的索引范围内时,也会引发 IndexError 错误。
为了保证程序的正确运行,可以在代码中添加一些异常处理机制来处理这些错误:
```
try:
a = input()
c = ['Math', 'Python Programming', 'Food science', 'Chinese']
for i in a:
d = c[int(i) - 1]
print(d)
except ValueError:
print("请输入一个数字!")
except IndexError:
print("输入的数字超出了范围!")
except:
print("程序运行错误!")
```
这样就可以保证程序在运行时能够正确处理各种异常情况,避免程序因错误而崩溃。
相关问题
c语言T = int(input())for _ in range(T): n = int(input()) students = [] for i in range(n): s, d = input().split() students.append((s, int(d))) students.sort(key=lambda x: (-x[1], x[0])) for i in range(n): print(i+1, students[i][0], students[i][1])
这段代码是Python代码,如果需要转换为C语言代码,需要进行以下修改:
1. 使用 `scanf()` 函数代替 `input()` 函数读取输入。
2. 使用结构体数组代替元组列表存储学生信息。
3. 使用 `qsort()` 函数代替 `sort()` 方法进行排序。
4. 输出结果时使用 `printf()` 函数代替 `print()` 函数。
修改后的C语言代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct student {
char s[9];
int d;
};
int cmp(const void *a, const void *b) {
struct student *s1 = (struct student *)a;
struct student *s2 = (struct student *)b;
if (s1->d != s2->d) {
return s2->d - s1->d;
} else {
return strcmp(s1->s, s2->s);
}
}
int main() {
int T;
scanf("%d", &T);
while (T--) {
int n;
scanf("%d", &n);
struct student students[100];
for (int i = 0; i < n; i++) {
scanf("%s%d", students[i].s, &students[i].d);
}
qsort(students, n, sizeof(struct student), cmp);
for (int i = 0; i < n; i++) {
printf("%d %s %d\n", i+1, students[i].s, students[i].d);
}
}
return 0;
}
```
首先读入测试数据组数 `T`,然后进行 `T` 次循环。每次循环首先读入学生总数 `n`,然后读入每个学生的信息,存储为一个结构体。
接下来使用 `qsort()` 函数对学生数组进行排序,排序时先按照解题总数 `d` 降序排序,如果 `d` 相同则按照学号 `s` 升序排序。这里使用了 `cmp()` 函数来指定排序规则。
最后循环输出每个学生的排名、学号和解题总数,排名从 1 开始递增。
将本段python代码转为c语言代码: n = int(input()) necklace = input() count = 0 for i in range(n): color = necklace[i % n] if color == 'w': count += 1 else: j = i while necklace[j % n] == color: j += 1 count += j - i print(count)
以下是将给定的 Python 代码转换成 C 语言代码的过程:
```c
#include <stdio.h>
#include <string.h>
int main() {
int n, count = 0, i, j;
char necklace[100000];
scanf("%d", &n);
scanf("%s", necklace);
for (i = 0; i < n; i++) {
char color = necklace[i % n];
if (color == 'w') {
count++;
} else {
j = i;
while (necklace[j % n] == color) {
j++;
}
count += j - i;
}
}
printf("%d\n", count);
return 0;
}
```
注意,这里我们假设项链字符串的最大长度为 100000。
阅读全文
相关推荐
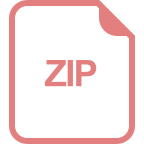
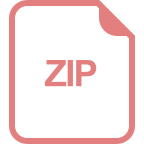
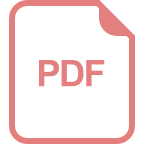














