代码如何使用PyTorch中的内存管理工具来优化内存的使用
时间: 2024-01-24 17:17:25 浏览: 21
在PyTorch中,内存管理的主要工具是torch.cuda.empty_cache()和torch.autograd.profiler.profile()。
1. torch.cuda.empty_cache():这个函数会清除PyTorch张量缓存中的所有未被引用的缓存。这些缓存可能会导致内存占用过高,因此可以使用该函数来释放这些缓存。
2. torch.autograd.profiler.profile():这个函数可以用来分析PyTorch代码的运行时间和内存使用情况。它可以帮助您找出哪些部分的代码使用了大量内存,以便进行优化。
以下是使用这些工具来优化内存使用的示例代码:
```python
import torch
import torch.autograd.profiler as profiler
# 定义一个简单的神经网络
class Net(torch.nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = torch.nn.Linear(10, 20)
self.fc2 = torch.nn.Linear(20, 1)
def forward(self, x):
x = self.fc1(x)
x = torch.nn.functional.relu(x)
x = self.fc2(x)
return x
# 创建一个模型实例和一些随机输入数据
model = Net()
inputs = torch.randn(1000, 10)
# 使用torch.autograd.profiler.profile()来分析模型的内存使用情况
with profiler.profile(record_shapes=True) as prof:
outputs = model(inputs)
# 打印分析结果
print(prof.key_averages().table(sort_by="self_cpu_memory_usage", row_limit=10))
# 清除PyTorch张量缓存中的所有未被引用的缓存
torch.cuda.empty_cache()
```
在上面的示例中,我们定义了一个简单的神经网络,并使用torch.autograd.profiler.profile()来分析其内存使用情况。然后,我们打印了使用内存最多的前10个操作的列表。最后,我们使用torch.cuda.empty_cache()来清除PyTorch张量缓存中的所有未被引用的缓存,以释放内存。
相关推荐
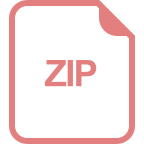
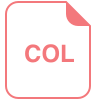
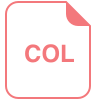
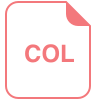
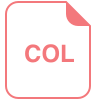
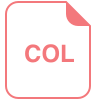









