用python写一个斗地主游戏
时间: 2024-05-29 07:03:39 浏览: 110
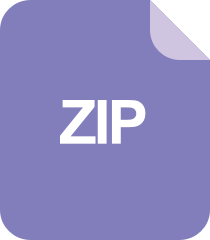
Python编写的斗地主
好的,我可以给你提供一个基本的代码框架,你可以在此基础上进行改进和完善。
```python
import random
class Card:
def __init__(self, suit, rank):
self.suit = suit
self.rank = rank
def __str__(self):
return self.suit + self.rank
class Deck:
def __init__(self):
self.cards = []
for suit in ['♠', '♥', '♣', '♦']:
for rank in ['2', '3', '4', '5', '6', '7', '8', '9', '10', 'J', 'Q', 'K', 'A']:
card = Card(suit, rank)
self.cards.append(card)
def shuffle(self):
random.shuffle(self.cards)
def deal(self):
return self.cards.pop()
class Player:
def __init__(self, name):
self.name = name
self.hand = []
def add_card(self, card):
self.hand.append(card)
def remove_card(self, card):
self.hand.remove(card)
def sort_hand(self):
self.hand.sort(key=lambda x: ('♠♥♣♦'.index(x.suit), '2345678910JQKA'.index(x.rank)))
def show_hand(self):
print(f"{self.name}'s hand:")
for card in self.hand:
print(card, end=' ')
print()
class Game:
def __init__(self):
self.deck = Deck()
self.deck.shuffle()
self.players = [Player('Player 1'), Player('Player 2'), Player('Player 3')]
self.landlord = None
self.current_player = None
def deal_cards(self):
for i in range(17):
for player in self.players:
card = self.deck.deal()
player.add_card(card)
for i in range(3):
card = self.deck.deal()
self.players[0].add_card(card)
def choose_landlord(self):
self.landlord = self.players[random.randint(0, 2)]
self.current_player = self.landlord
print(f"{self.landlord.name} is the landlord!")
def next_player(self):
index = self.players.index(self.current_player)
self.current_player = self.players[(index + 1) % 3]
def play(self):
self.deal_cards()
self.choose_landlord()
for player in self.players:
player.sort_hand()
player.show_hand()
while True:
if len(self.players[0].hand) == 0:
print(f"{self.players[0].name} wins!")
break
if len(self.players[1].hand) == 0 or len(self.players[2].hand) == 0:
print(f"{self.landlord.name} wins!")
break
if self.current_player == self.players[0]:
print("It's your turn!")
self.players[0].show_hand()
# TODO: allow the player to play cards
else:
print(f"{self.current_player.name}'s turn")
# TODO: implement AI for other players
# Play cards according to the rules of the game
self.next_player()
if __name__ == '__main__':
game = Game()
game.play()
```
以上代码实现了一个简单的斗地主游戏,其中AI部分还需要你自己去实现。你可以通过添加函数和修改代码来完成游戏。
阅读全文
相关推荐
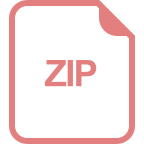















