if(level>last_level) { level_change=1; sprintf((char*)str_level,"A:H%d+L%d+U\r\n",height,level); HAL_UART_Transmit(&huart1,str_level,sizeof(str_level),50); } else if(level<last_level) { level_change=1; sprintf((char*)str_level,"A:H%d+L%d+D\r\n",height,level); HAL_UART_Transmit(&huart1,str_level,sizeof(str_level),50); } }
时间: 2023-09-11 15:07:52 浏览: 105
这段代码看起来像是在控制某个系统的高度和水平等级,其中使用了串口通信。变量 level 和 last_level 用来进行比较,如果 level 大于 last_level,则会发送一个包含高度和水平等级的信息并且状态为上升;如果 level 小于 last_level,则会发送一个包含高度和水平等级的信息并且状态为下降。在这段代码中还有其他的变量和函数调用,可能需要更多上下文才能够完全理解其功能。
相关问题
优化一下代码static void fill_file_chunk(const char *path, int *number, int level) { DIR *dir = NULL; struct dirent *entry = NULL; int i = 0; ++level; if(level > 3) return; dir = opendir(path); if(dir == NULL) return; while((entry = readdir(dir)) != NULL) { if(strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) continue; if(entry->d_type == 4 && level == 1){ if(check_dir_name(entry->d_name) == 0) continue; char buffer[MAX_PATH]; sprintf(buffer, "%s/%s", path, entry->d_name); fill_file_chunk(buffer, number, level); } if(entry->d_type == 4 && level == 2){ if(check_dir_name(entry->d_name) == 0) continue; char buffer[MAX_PATH]; sprintf(buffer, "%s/%s", path, entry->d_name); fill_file_chunk(buffer, number, level); } if(entry->d_type == 8 && level ==3){ if(check_file_name(entry->d_name) == 0) continue; if(*number == max_file_chunk){ file_chunks = (struct FileChunk *)realloc(file_chunks, (max_file_chunk + FILE_CHUNK_INCREAMENT) * sizeof(struct FileChunk)); if(file_chunks == NULL) break; max_file_chunk += FILE_CHUNK_INCREAMENT; } char buffer[MAX_PATH]; sprintf(buffer, "%s/%s", path, entry->d_name); i = *number; strcpy(file_chunks[i].file_name, entry->d_name); sprintf(file_chunks[i].file_path, buffer); file_chunks[i].file_size = get_file_size(buffer); *number = i + 1; } else continue; } closedir(dir); return; }
Here are some suggested optimizations for the given code:
1. Use switch case instead of if-else: In the current code, multiple if-else statements are being used to check for different conditions. This can be optimized by using switch case instead, which can improve readability and maintainability of the code.
2. Use early return to reduce nested if-else: In the current code, multiple if-else statements are nested inside a while loop, which can make the code difficult to read and understand. By using early return, we can reduce the level of nesting and make the code more readable.
3. Avoid redundant code: In the current code, there are multiple instances where the same block of code is being repeated. This can be optimized by refactoring the code and avoiding the duplication of code.
Here is the optimized code:
```
static void fill_file_chunk(const char *path, int *number, int level) {
DIR *dir = opendir(path);
if(dir == NULL) {
return;
}
++level;
if(level > 3) {
closedir(dir);
return;
}
struct dirent *entry;
while((entry = readdir(dir)) != NULL) {
switch(entry->d_type) {
case DT_DIR:
if(level == 1 || level == 2) {
if(check_dir_name(entry->d_name) == 0) {
continue;
}
char buffer[MAX_PATH];
sprintf(buffer, "%s/%s", path, entry->d_name);
fill_file_chunk(buffer, number, level);
}
break;
case DT_REG:
if(level == 3) {
if(check_file_name(entry->d_name) == 0) {
continue;
}
if(*number == max_file_chunk) {
file_chunks = (struct FileChunk *)realloc(file_chunks, (max_file_chunk + FILE_CHUNK_INCREAMENT) * sizeof(struct FileChunk));
if(file_chunks == NULL) {
break;
}
max_file_chunk += FILE_CHUNK_INCREAMENT;
}
char buffer[MAX_PATH];
sprintf(buffer, "%s/%s", path, entry->d_name);
int i = *number;
strcpy(file_chunks[i].file_name, entry->d_name);
sprintf(file_chunks[i].file_path, buffer);
file_chunks[i].file_size = get_file_size(buffer);
*number = i + 1;
}
break;
default:
break;
}
}
closedir(dir);
}
```
This optimized code uses switch case instead of if-else statements, reduces the level of nesting by using early return, and avoids redundant code by refactoring the code.
if(huart->Instance==USART1) { if(str_rx[0]=='C') { rx_flag=1; sprintf((char*)str_tx1,"C:H%d+L%d\r\n",height,level); HAL_UART_Transmit(&huart1,str_tx1,sizeof(str_tx1),50); } else if(str_rx[0]=='S') { rx_flag=1; sprintf((char*)str_tx2,"S:TL%d+TL%d+TL%d\r\n",Threshold1,Threshold2,Threshold3); HAL_UART_Transmit(&huart1,str_tx2,sizeof(str_tx2),50); } } HAL_UART_Receive_IT(huart,str_rx,1);
这是一个串口接收中断的处理函数。该函数的作用是检测串口接收到的数据,并根据不同的数据进行不同的处理。
首先,判断当前串口实例是否为 USART1。如果是,则根据接收到的数据进行处理。如果接收到的数据的第一个字符是 'C',则将 rx_flag 标志位设置为 1,并使用 sprintf 函数构造一个包含 height 和 level 值的字符串,并通过 USART1 发送出去。如果接收到的数据的第一个字符是 'S',则将 rx_flag 标志位设置为 1,并使用 sprintf 函数构造一个包含 Threshold1、Threshold2 和 Threshold3 值的字符串,并通过 USART1 发送出去。
接着,调用 HAL 库的函数,重新启动串口接收中断,以便继续接收数据。
需要注意的是,该代码中的 HAL_UART_Receive_IT 函数使用了中断方式进行串口数据接收,因此在主程序中需要先使能相应串口的中断功能。
阅读全文
相关推荐
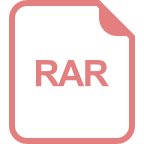
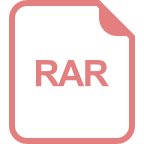
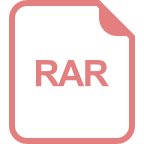














