java创建双向链表_Java双向链表创建/输出---基础<Base>:
时间: 2024-05-14 08:19:25 浏览: 100
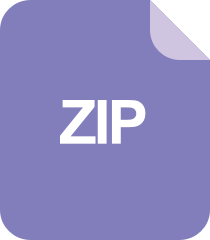
doublelink.zip_DoubleLink java_双向链表实现
双向链表是一种常见的数据结构,与单向链表相比,它可以在节点之间进行双向遍历。在Java中,我们可以使用类来实现双向链表。
下面是一个简单的Java程序,演示如何创建和输出双向链表:
```java
// 双向链表节点类
class Node {
public int data;
public Node prev;
public Node next;
public Node(int data) {
this.data = data;
this.prev = null;
this.next = null;
}
}
// 双向链表类
class DoubleLinkedList {
public Node head;
public Node tail;
public DoubleLinkedList() {
this.head = null;
this.tail = null;
}
// 在链表头部插入节点
public void insertAtHead(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
tail = newNode;
return;
}
newNode.next = head;
head.prev = newNode;
head = newNode;
}
// 在链表尾部插入节点
public void insertAtTail(int data) {
Node newNode = new Node(data);
if (tail == null) {
head = newNode;
tail = newNode;
return;
}
newNode.prev = tail;
tail.next = newNode;
tail = newNode;
}
// 输出链表
public void printList() {
Node current = head;
while (current != null) {
System.out.print(current.data + " ");
current = current.next;
}
System.out.println();
}
}
// 测试类
public class Main {
public static void main(String[] args) {
DoubleLinkedList list = new DoubleLinkedList();
list.insertAtHead(1);
list.insertAtHead(2);
list.insertAtTail(3);
list.insertAtTail(4);
list.printList(); // 输出:2 1 3 4
}
}
```
在上面的程序中,我们首先定义了一个`Node`类来表示双向链表的每个节点,其中包含了当前节点的值、前一个节点和后一个节点。接着,我们定义了`DoubleLinkedList`类来表示整个双向链表,其中包含了头节点和尾节点。
在`DoubleLinkedList`类中,我们定义了`insertAtHead`和`insertAtTail`方法来在链表头部和尾部插入节点,分别需要创建一个新节点,并将它与当前链表的头节点或尾节点进行连接。
最后,我们定义了`printList`方法来输出整个链表,只需要从链表的头节点开始遍历,依次输出每个节点的值即可。
在`Main`类中,我们通过`DoubleLinkedList`类创建了一个双向链表,并向其中插入了四个节点。最后,我们调用`printList`方法输出整个链表。
阅读全文
相关推荐
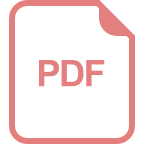
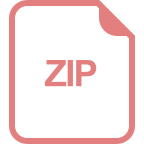
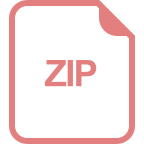
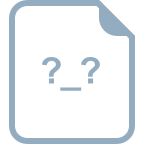
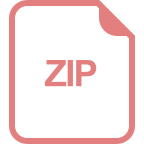
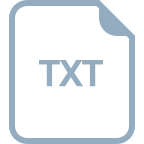
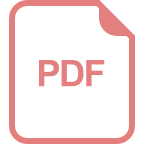
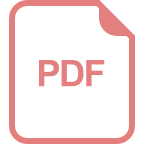
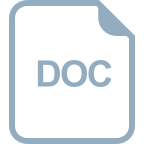
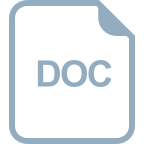
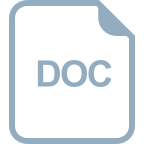
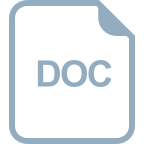
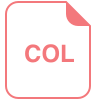
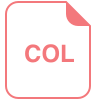
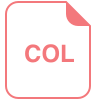
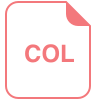