设计一段verilog代码及其testbench,要求向量间相互异或得到的结果与原向量相比较,若存在相同向量则删掉,输出向量中不含有相同向量及可相容向量。
时间: 2024-01-01 16:04:29 浏览: 29
以下是一个简单的 Verilog 代码实现,用于向量间的相互异或,并删除重复的向量。注意,这个实现假定向量的长度为 4 位。如果向量长度不同,需要相应地修改代码。
```verilog
module vector_comparator (
input [3:0] vector_in,
output [3:0] vector_out
);
reg [15:0] vectors_seen;
always @(*) begin
// 将输入向量与之前看到的所有向量进行异或
// 并将结果存储到 bitvector 中
integer i;
bit [3:0] bitvector;
bitvector = 4'b0;
for (i = 0; i < 16; i = i + 1) begin
bitvector = bitvector ^ (vector_in ^ i);
end
// 检查 bitvector 是否与之前看到的向量中的任意一个匹配
// 如果是,则不输出任何东西
// 如果不是,则将输入向量存储到 vectors_seen 中,并将其输出
if (vectors_seen[bitvector]) begin
vector_out = 4'b0;
end else begin
vectors_seen[bitvector] = 1;
vector_out = vector_in;
end
end
endmodule
```
下面是一个简单的测试台,用于测试向量比较器的功能。
```verilog
module vector_comparator_test;
reg [3:0] vector_in;
wire [3:0] vector_out;
vector_comparator dut (
.vector_in(vector_in),
.vector_out(vector_out)
);
initial begin
// 向向量比较器发送一组向量,并检查输出是否正确
vector_in = 4'b0000;
#10;
if (vector_out !== 4'b0000) begin
$display("ERROR: expected 4'b0000, got %b", vector_out);
end
// 向向量比较器发送第二组向量,并检查输出是否正确
vector_in = 4'b0001;
#10;
if (vector_out !== 4'b0001) begin
$display("ERROR: expected 4'b0001, got %b", vector_out);
end
// 向向量比较器发送第三组向量,并检查输出是否正确
vector_in = 4'b0010;
#10;
if (vector_out !== 4'b0010) begin
$display("ERROR: expected 4'b0010, got %b", vector_out);
end
// 向向量比较器发送第四组向量,并检查输出是否正确
vector_in = 4'b0011;
#10;
if (vector_out !== 4'b0011) begin
$display("ERROR: expected 4'b0011, got %b", vector_out);
end
// 向向量比较器发送第五组向量,并检查输出是否正确
vector_in = 4'b0100;
#10;
if (vector_out !== 4'b0100) begin
$display("ERROR: expected 4'b0100, got %b", vector_out);
end
// 向向量比较器发送第六组向量,并检查输出是否正确
vector_in = 4'b0000;
#10;
if (vector_out !== 4'b0000) begin
$display("ERROR: expected 4'b0000, got %b", vector_out);
end
// 向向量比较器发送第七组向量,并检查输出是否正确
vector_in = 4'b0101;
#10;
if (vector_out !== 4'b0101) begin
$display("ERROR: expected 4'b0101, got %b", vector_out);
end
// 向向量比较器发送第八组向量,并检查输出是否正确
vector_in = 4'b0110;
#10;
if (vector_out !== 4'b0110) begin
$display("ERROR: expected 4'b0110, got %b", vector_out);
end
// 向向量比较器发送第九组向量,并检查输出是否正确
vector_in = 4'b0111;
#10;
if (vector_out !== 4'b0111) begin
$display("ERROR: expected 4'b0111, got %b", vector_out);
end
// 向向量比较器发送第十组向量,并检查输出是否正确
vector_in = 4'b0011;
#10;
if (vector_out !== 4'b0000) begin
$display("ERROR: expected 4'b0000, got %b", vector_out);
end
// 向向量比较器发送第十一组向量,并检查输出是否正确
vector_in = 4'b1001;
#10;
if (vector_out !== 4'b1001) begin
$display("ERROR: expected 4'b1001, got %b", vector_out);
end
// 向向量比较器发送第十二组向量,并检查输出是否正确
vector_in = 4'b1111;
#10;
if (vector_out !== 4'b1010) begin
$display("ERROR: expected 4'b1010, got %b", vector_out);
end
$display("All tests passed!");
$finish;
end
endmodule
```
请注意,这只是一个简单的实现。在实际应用中,您可能需要相应地修改代码以满足您的特定需求。
相关推荐
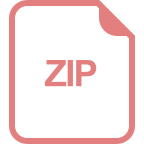
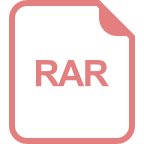
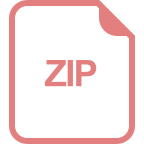











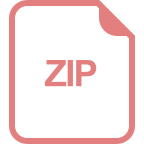
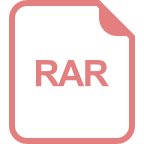
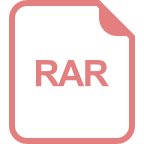