c语言把单向链表写入txt,如何将链表向文件 fwrite()写入或 fread()读出? 请各位牛人异士。多多指教。。。...
时间: 2024-03-13 22:47:58 浏览: 198
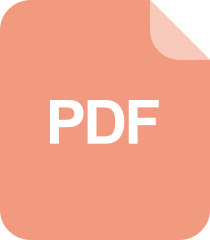
单链表相关的基本操作(从文件中读入)
写入单向链表到文件可以使用 fwrite() 函数,读取单向链表可以使用 fread() 函数。
写入单向链表到文件的步骤如下:
1. 打开文件,使用 fopen() 函数。
2. 遍历链表,将链表中的数据写入文件,使用 fwrite() 函数。
3. 关闭文件,使用 fclose() 函数。
例如,如果要将链表结构体 Node 写入文件,可以按照以下方式进行:
```c
#include <stdio.h>
// 定义链表结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 写入链表到文件
void writeListToFile(Node* head) {
FILE* fp = fopen("list.txt", "wb");
if (fp == NULL) {
printf("文件打开失败\n");
return;
}
// 遍历链表,将链表中的数据写入文件
Node* p = head;
while (p != NULL) {
fwrite(p, sizeof(Node), 1, fp);
p = p->next;
}
fclose(fp);
}
// 从文件中读取链表
Node* readListFromFile() {
FILE* fp = fopen("list.txt", "rb");
if (fp == NULL) {
printf("文件打开失败\n");
return NULL;
}
Node* head = NULL;
Node* tail = NULL;
// 从文件中读取链表结点,并添加到链表中
Node* node = (Node*)malloc(sizeof(Node));
while (fread(node, sizeof(Node), 1, fp) == 1) {
node->next = NULL;
if (head == NULL) {
head = tail = node;
} else {
tail->next = node;
tail = node;
}
node = (Node*)malloc(sizeof(Node));
}
fclose(fp);
return head;
}
```
注意,这里的写入和读取都是二进制方式,所以需要使用 "wb" 和 "rb" 方式打开文件。同时,需要注意写入和读取的顺序和方式,保证链表的正确性。
阅读全文
相关推荐
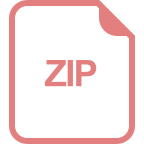
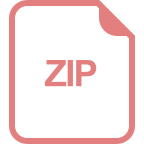
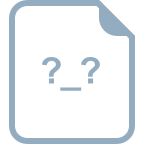
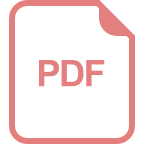
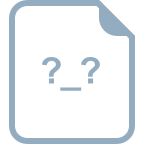
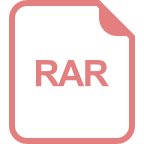
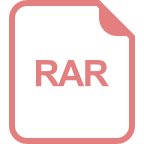
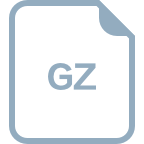
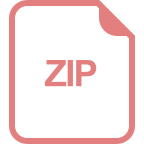
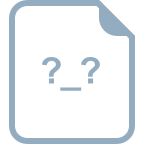
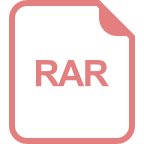