A shop uses a class to store data about items for sale in the shop. This data includes: name of item (for example: “bread”) price of item (for example: $3.19) number of items in stock (for example: 120) Design the class and write suitable methods to load the data and display the data. There are many items in the shop so you will need an array of objects. Write a method that enters the name of an item and how many items have just been sold. The method must also update the number of items in the object. for example: Enter item that was just sold: bread How many items were sold: 2 Number of bread items in the shop is now 118 Write a main program to test your class. 使用c++,要求自己输入物品价格名称等
时间: 2024-01-21 14:01:58 浏览: 56
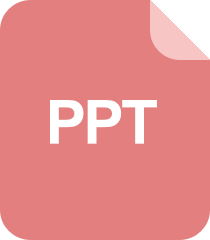
有关对象操作语句-JavaScript教程
以下是使用 C++ 实现的代码:
```c++
#include <iostream>
#include <string>
using namespace std;
class Item {
public:
string name;
double price;
int stock;
void display() {
cout << "Name: " << name << endl;
cout << "Price: $" << price << endl;
cout << "Stock: " << stock << endl;
}
void sell(int num) {
stock -= num;
cout << "Number of " << name << " items in the shop is now " << stock << endl;
}
};
int main() {
const int NUM_ITEMS = 3;
Item items[NUM_ITEMS];
for (int i = 0; i < NUM_ITEMS; i++) {
cout << "Enter name of item " << i+1 << ": ";
getline(cin, items[i].name);
cout << "Enter price of item " << i+1 << ": $";
cin >> items[i].price;
cout << "Enter number of items in stock for item " << i+1 << ": ";
cin >> items[i].stock;
cin.ignore(); // Ignore newline character
cout << endl;
}
for (int i = 0; i < NUM_ITEMS; i++) {
items[i].display();
cout << endl;
}
string item_name;
int num_sold;
cout << "Enter item that was just sold: ";
getline(cin, item_name);
cout << "How many items were sold: ";
cin >> num_sold;
for (int i = 0; i < NUM_ITEMS; i++) {
if (items[i].name == item_name) {
items[i].sell(num_sold);
break;
}
}
return 0;
}
```
在这个程序中,我们定义了一个名为 `Item` 的类,其中包含了物品的名称、价格和库存量,并定义了两个方法:`display()` 用于显示物品的信息,`sell()` 用于出售物品并更新库存量。在 `main()` 函数中,我们创建了一个大小为 `NUM_ITEMS` 的 `Item` 对象数组,并向用户询问每个物品的信息。然后,我们遍历数组并显示每个物品的信息。最后,我们要求用户输入出售的物品和数量,并在数组中查找该物品并出售,最后更新库存量。
阅读全文
相关推荐
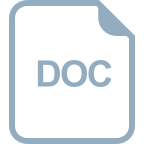
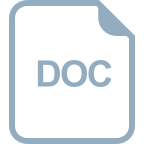
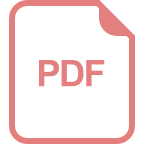
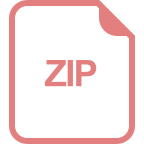
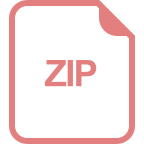
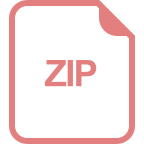
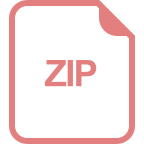
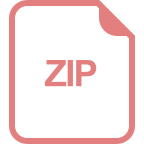
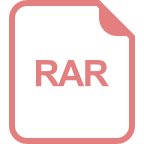
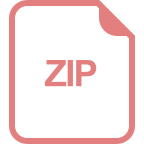
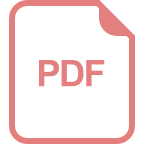
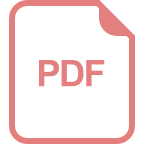
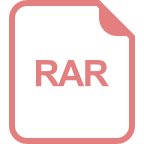
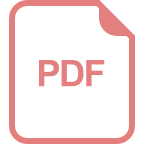
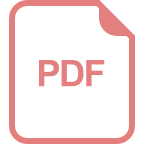
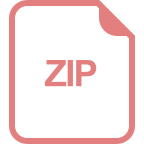
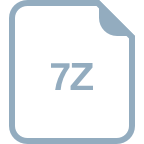