写一个包含CreateThread 函数的cpp
时间: 2023-01-30 18:20:34 浏览: 196
下面是一个包含CreateThread函数的简单示例代码:
```cpp
#include <windows.h>
#include <iostream>
using namespace std;
// 线程函数,在新建的线程中执行
DWORD WINAPI thread_func(LPVOID lpParameter) {
cout << "Hello from the new thread!" << endl;
return 0;
}
int main() {
// 创建新线程
HANDLE hThread = CreateThread(NULL, 0, thread_func, NULL, 0, NULL);
if (hThread == NULL) {
cout << "Failed to create thread." << endl;
return -1;
}
cout << "Hello from the main thread." << endl;
// 等待新线程结束
WaitForSingleObject(hThread, INFINITE);
// 关闭线程句柄
CloseHandle(hThread);
return 0;
}
```
这段代码会在主线程中输出 "Hello from the main thread.",并在新建的线程中输出 "Hello from the new thread!"。
相关问题
QT CreateThread函数
QT中的`QThread`是一个用于创建独立线程的工具,它提供了一种在Qt应用程序中管理并发执行任务的方式。`CreateThread`并不是QT直接提供的函数,但在使用`QThread`时,你可以通过创建一个新的`QThread`实例,并在其构造函数中设置线程函数(`moveToThread`)来启动一个新的线程。
例如:
```cpp
#include <QObject>
#include <QThread>
class Worker : public QObject {
public:
void run() {
// 这里放置你需要在新线程上执行的任务
qDebug() << "Worker thread running";
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// 创建工作线程
Worker worker;
QThread* thread = new QThread;
// 将Worker对象移动到新线程
connect(thread, &QThread::started, &worker, [this] { this->moveToThread(thread); });
connect(&worker, &QObject::finished, thread, &QThread::quit);
connect(thread, &QThread::finished, &worker, &QObject::deleteLater);
connect(thread, &QThread::finished, thread, &QThread::deleteLater);
// 启动新线程
thread->start();
return app.exec();
}
```
在这个例子中,`thread->start()`会开始新线程并运行`run()`函数。
SD_CreateThread函数
SD_CreateThread是一个假设性的函数名,它通常出现在某些操作系统或者SDK(Software Development Kit)中,用于创建线程。在Windows API(例如微软的Windows编程接口)中,可能会有类似`CreateThread`这样的函数,它的作用是用来启动一个新的线程,这个线程可以执行独立于主线程的任务。
这个函数的基本原型可能是这样的:
```cpp
HANDLE SD_CreateThread(
LPSECURITY_ATTRIBUTES lpThreadAttributes,
SIZE_T dwStackSize,
LPTHREAD_START_ROUTINE lpStartAddress,
PVOID lpParameter,
DWORD dwCreationFlags,
LPDWORD lpThreadId
);
```
参数含义如下:
- `lpThreadAttributes`: 线程属性指针,可以设置线程的一些特性。
- `dwStackSize`: 新线程堆栈大小。
- `lpStartAddress`: 要执行的线程启动函数的地址。
- `lpParameter`: 传递给启动函数的参数。
- `dwCreationFlags`: 创建标志,控制线程的行为和优先级等。
- `lpThreadId`: 可选返回值,新线程的ID。
使用这个函数后,你需要通过`WaitForSingleObject`等待线程完成,或者使用`CloseHandle`关闭不再需要的线程句柄。
阅读全文
相关推荐
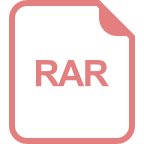
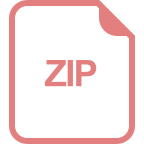
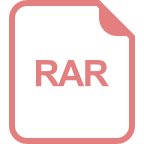













