fastapi中post请求上传的图片,用tesseract对图片进行转文字并输出
时间: 2023-08-21 19:04:34 浏览: 44
要在 FastAPI 中使用 Tesseract 对上传的图片进行 OCR(光学字符识别)并输出文字,你可以按照以下步骤进行操作:
首先,确保已经安装了 Tesseract OCR。你可以通过以下命令在终端中安装 Tesseract:
```
sudo apt-get install tesseract-ocr
```
在 Python 中,可以使用 pytesseract 库来调用 Tesseract。确保已经安装了 pytesseract:
```
pip install pytesseract
```
接下来,你需要使用 PIL(Python Imaging Library)库来处理上传的图片。确保已经安装了 PIL:
```
pip install pillow
```
然后,你可以按照以下步骤在 FastAPI 中进行处理:
1. 导入所需的模块和库:
```python
from fastapi import FastAPI, UploadFile, File
from PIL import Image
import pytesseract
app = FastAPI()
```
2. 定义一个路由来接受 POST 请求,并声明需要接受文件上传:
```python
@app.post("/upload-image/")
async def upload_image(image: UploadFile = File(...)):
# 保存上传的图片到临时文件
file_path = f"temp/{image.filename}"
with open(file_path, "wb") as f:
f.write(await image.read())
# 使用 PIL 打开图片
pil_image = Image.open(file_path)
# 使用 pytesseract 进行 OCR
text = pytesseract.image_to_string(pil_image)
# 返回识别的文字
return {"text": text}
```
3. 在处理函数中,首先将上传的图片保存到临时文件中。然后,使用 PIL 的 `Image.open()` 方法打开图片。
4. 使用 pytesseract 的 `image_to_string()` 方法对图片进行 OCR,并将结果保存在 `text` 变量中。
5. 最后,通过返回一个 JSON 对象来提供识别的文字。
这样,当你向 `/upload-image/` 路由发送 POST 请求时,FastAPI 将会接受上传的图片,使用 Tesseract 对其进行 OCR,并返回识别的文字。
相关推荐
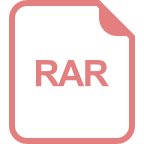













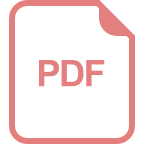