C++中explicit operator type();和operator type();的区别
时间: 2024-05-15 09:14:33 浏览: 9
`explicit operator type();` 声明了一个显式类型转换运算符,而 `operator type();` 声明了一个隐式类型转换运算符。
显式类型转换运算符只能通过显式地调用来进行类型转换,例如:
```cpp
class Foo {
public:
explicit operator int() const { return 42; }
};
int main() {
Foo foo;
int i = static_cast<int>(foo); // 显式调用转换运算符
}
```
而隐式类型转换运算符则可以在需要进行类型转换时自动调用,例如:
```cpp
class Bar {
public:
operator int() const { return 42; }
};
int main() {
Bar bar;
int i = bar; // 隐式调用转换运算符
}
```
需要注意的是,显式类型转换运算符并不会禁止隐式类型转换,而是只能通过显式调用来进行类型转换。而隐式类型转换运算符则可能会导致意外的类型转换,因此应该谨慎使用。
相关问题
解释关键字 explicit operator
`explicit operator` 是 C++ 中的一个关键字,用于定义用户自定义类型转换。通常情况下,C++ 中的类型转换是隐式的,即编译器会自动将一种类型转换为另一种类型,而程序员无需显式地指定。但有时我们需要显式地定义类型转换,以确保类型转换的行为符合我们的期望。这时就可以使用 `explicit operator`。
例如,我们定义了一个名为 `MyClass` 的类,并希望能够将其转换为 `int` 类型。我们可以在 `MyClass` 类中定义一个 `explicit` 的类型转换函数,如下所示:
```
class MyClass {
public:
explicit operator int() const {
return 0; // 假设我们要将 MyClass 转换为 int 类型,此处返回一个固定值 0
}
};
```
这样,如果我们需要将 `MyClass` 类型的对象转换为 `int` 类型,就必须显式地调用类型转换函数,例如:
```
MyClass obj;
int val = static_cast<int>(obj);
```
这里使用了 `static_cast` 进行显式转换,因为类型转换函数标记为 `explicit`,不能进行隐式转换。通过这种方式,程序员可以更加精确地控制类型转换的行为,避免出现不必要的错误。
C++operator bool
引用\[1\]中的代码展示了一个C++程序,其中定义了两个结构体A和B,并重载了它们的bool和!运算符。在main函数中,创建了A对象a和B对象b,并使用!运算符对它们进行判断。引用\[2\]中的代码也展示了一个C++程序,其中只定义了一个结构体A,并重载了它的bool运算符。在main函数中,创建了A对象a,并使用!运算符对它进行判断。引用\[3\]中提到了一个函数explicit operator bool() const,它是一种运算符重载,用于将本类型对象隐式转换为bool类型。
回答: 在C++中,operator bool是一种运算符重载,用于将对象转换为bool类型。在引用\[1\]中的代码中,结构体A和B都重载了operator bool运算符,分别用于判断对象a和b的bool值。在引用\[2\]中的代码中,结构体A也重载了operator bool运算符,用于判断对象a的bool值。而引用\[3\]中提到的explicit operator bool() const是一种显式转换函数,用于将本类型对象隐式转换为bool类型。
#### 引用[.reference_title]
- *1* *2* [C++ operator bool](https://blog.csdn.net/weixin_30326745/article/details/99955254)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [【C++】operator bool() 和 operator const bool() const](https://blog.csdn.net/u010168781/article/details/95645564)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
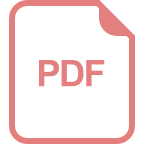
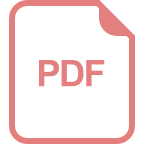
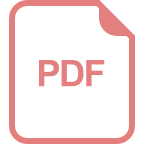












