分别用文本文件和CSV文件保存5个学生的课程成绩。编写一个程序,从文件读取成绩,计算总分,按总分按从高到低的顺序输出。
时间: 2024-02-17 13:02:48 浏览: 149
下面是一个Python程序,可以从文本文件和CSV文件中读取学生成绩并按总分排序输出:
```python
import csv
# 从文本文件中读取成绩
def read_txt_file(filename):
scores = {}
with open(filename, 'r') as f:
for line in f:
name, score = line.strip().split(',')
scores[name] = [int(score)]
return scores
# 从CSV文件中读取成绩
def read_csv_file(filename):
scores = {}
with open(filename, 'r') as f:
reader = csv.reader(f)
for row in reader:
name, score = row
scores[name] = [int(score)]
return scores
# 计算总分
def calculate_total(scores):
for name in scores:
score = scores[name][0]
total = sum(score)
scores[name].append(total)
# 按总分排序输出
def sort_by_total(scores):
sorted_scores = sorted(scores.items(), key=lambda x: x[1][1], reverse=True)
for name, score in sorted_scores:
print('{}: {}'.format(name, score[1]))
# 从文本文件读取成绩并计算总分
scores_txt = read_txt_file('scores.txt')
calculate_total(scores_txt)
# 从CSV文件读取成绩并计算总分
scores_csv = read_csv_file('scores.csv')
calculate_total(scores_csv)
# 按总分排序输出
sort_by_total(scores_txt)
sort_by_total(scores_csv)
```
其中,`scores.txt`和`scores.csv`文件分别保存了5个学生的成绩信息,格式如下:
```
张三,80
李四,90
王五,85
赵六,95
钱七,75
```
程序首先定义了三个函数,`read_txt_file()`函数从文本文件中读取成绩,`read_csv_file()`函数从CSV文件中读取成绩,`calculate_total()`函数计算每个学生的总分。然后,程序分别读取文本文件和CSV文件中的成绩信息,并计算总分。最后,程序调用`sort_by_total()`函数按总分排序输出学生信息。
阅读全文
相关推荐
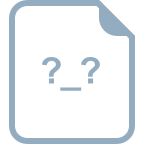
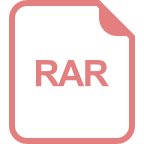
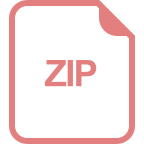














