cubic_interpolate() 如何实现
时间: 2023-11-24 13:07:11 浏览: 42
cubic_interpolate() 是一种插值方法,用于在给定一组数据点的情况下,通过三次函数来估计在两个数据点之间的值。其实现步骤如下:
1. 找到待插值的点所在的区间,并取出该区间内的四个数据点。
2. 根据这四个数据点,构建一个三次函数。
3. 将这个三次函数代入插值点的横坐标,计算出对应的纵坐标。
4. 返回计算得到的纵坐标,即为插值结果。
具体的实现代码如下:
```python
def cubic_interpolate(x, x0, y0, m0, x1, y1, m1):
"""
x: 待插值点的横坐标
x0, x1: 区间端点的横坐标
y0, y1: 区间端点的纵坐标
m0, m1: 区间端点的导数
"""
# 计算区间距离和方向
h = x1 - x0
t = (x - x0) / h
# 计算三次函数的系数
a = 2 * (y0 - y1) + h * (m0 + m1)
b = -3 * (y0 - y1) - h * (2 * m0 + m1)
c = m0
d = y0
# 计算插值结果
return a * t ** 3 + b * t ** 2 + c * t + d
```
需要注意的是,该函数要求输入的四个数据点必须在横坐标上严格单调递增,且插值点的横坐标必须在区间端点之间。如果插值点在区间外,或者数据点不满足单调递增的条件,则可能会得到错误的结果。
相关问题
cubic_interpolate() 如何实现三维插值
对于三维插值,我们需要使用三维网格来表示我们要插值的数据。假设我们有一个 $n\times m\times l$ 的三维网格,其中每个网格点上都有一个标量值 $f(x,y,z)$。现在我们想要在这个网格中找到一个任意位置 $(x_0, y_0, z_0)$ 上的值。
三维插值的基本思想是,首先找到离 $(x_0, y_0, z_0)$ 最近的八个网格点,然后利用这些网格点的值计算一个插值结果。最常用的三维插值方法是三线性插值,其基本思想是将三维插值问题分解成三个一维插值问题,然后对这三个方向分别进行线性插值。
具体来说,我们首先在 $x$ 方向上进行线性插值,计算出两个横向的插值结果 $f_{c_0,r_0,s_0}$ 和 $f_{c_1,r_0,s_0}$,其中 $c_0$ 和 $c_1$ 分别表示 $x_0$ 所在的两个网格点的列数,$r_0$ 和 $s_0$ 分别表示 $x_0$ 所在的两个网格点的行数和深度。具体地,我们有:
$$
f_{c_0,r_0,s_0} = f(c_0, r_0, s_0) \cdot (1-\alpha) + f(c_1, r_0, s_0) \cdot \alpha
$$
其中 $\alpha$ 是一个权重系数,满足 $0\leq\alpha\leq1$,其计算方式为:
$$
\alpha = \frac{x_0 - x_{c_0}}{x_{c_1} - x_{c_0}}
$$
其中 $x_{c_0}$ 和 $x_{c_1}$ 分别表示 $x_0$ 所在的两个网格点的列坐标。
接着,在 $y$ 方向上进行线性插值,计算出两个纵向的插值结果 $f_{c_0,r_1,s_0}$ 和 $f_{c_1,r_1,s_0}$,其计算方式与上面类似:
$$
f_{c_0,r_1,s_0} = f(c_0, r_1, s_0) \cdot (1-\beta) + f(c_1, r_1, s_0) \cdot \beta
$$
$$
\beta = \frac{y_0 - y_{r_0}}{y_{r_1} - y_{r_0}}
$$
最后,在 $z$ 方向上进行线性插值,计算出两个深度方向的插值结果 $f_{c_0,r_1,s_1}$ 和 $f_{c_1,r_1,s_1}$,其计算方式与上面类似:
$$
f_{c_0,r_1,s_1} = f(c_0, r_1, s_1) \cdot (1-\gamma) + f(c_1, r_1, s_1) \cdot \gamma
$$
$$
\gamma = \frac{z_0 - z_{s_0}}{z_{s_1} - z_{s_0}}
$$
最终的插值结果为:
$$
f(x_0, y_0, z_0) = f_{c_0,r_0,s_0} \cdot (1-\delta_x) \cdot (1-\delta_y) \cdot (1-\delta_z) + f_{c_1,r_0,s_0} \cdot \delta_x \cdot (1-\delta_y) \cdot (1-\delta_z) + f_{c_0,r_1,s_0} \cdot (1-\delta_x) \cdot \delta_y \cdot (1-\delta_z) + f_{c_1,r_1,s_0} \cdot \delta_x \cdot \delta_y \cdot (1-\delta_z) + f_{c_0,r_1,s_1} \cdot (1-\delta_x) \cdot \delta_y \cdot \delta_z + f_{c_1,r_1,s_1} \cdot \delta_x \cdot \delta_y \cdot \delta_z
$$
其中 $\delta_x = 1-\alpha$,$\delta_y = 1-\beta$,$\delta_z = 1-\gamma$。
interpolate well
根据提供的引用内容,我可以为您介绍一下如何进行插值以获得良好的结果。
插值是一种通过已知数据点来估计未知数据点的方法。在科学计算中,插值常用于填补缺失数据、平滑数据或生成连续函数。在Python中,scipy.interpolate模块提供了多种插值方法。
要获得良好的插值结果,可以考虑以下几点:
1. 选择合适的插值方法:scipy.interpolate模块提供了多种插值方法,如线性插值、多项式插值、样条插值等。根据数据的特点和需求,选择适合的插值方法可以获得更好的结果。
2. 确保数据点的分布均匀:插值方法对数据点的分布敏感,如果数据点过于密集或过于稀疏,都可能导致插值结果不准确。因此,在进行插值之前,可以考虑对数据进行预处理,使数据点的分布更加均匀。
3. 调整插值参数:某些插值方法可能有一些参数可以调整,通过调整这些参数,可以进一步优化插值结果。可以根据具体的插值方法文档或实验来选择合适的参数值。
以下是一个使用scipy.interpolate模块进行插值的示例:
```python
from scipy.interpolate import interp1d
# 假设有一组已知数据点
x = [1, 2, 3, 4, 5]
y = [2, 4, 1, 6, 3]
# 创建插值函数
f = interp1d(x, y, kind='cubic')
# 在新的x值上进行插值
new_x = [1.5, 2.5, 3.5]
new_y = f(new_x)
print(new_y) # 输出插值结果
```
这是一个简单的一维插值示例,使用了scipy.interpolate模块中的interp1d函数进行插值。在这个示例中,我们通过已知的数据点(x, y)创建了一个插值函数f,并在新的x值上进行插值,得到了新的y值。
相关推荐
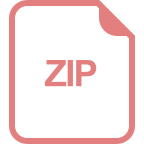
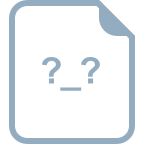
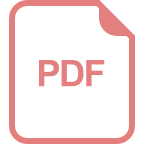












