python实现PageRank算法
时间: 2023-02-16 13:28:57 浏览: 209
PageRank算法是Google搜索算法的核心算法之一。它通过对网页之间的链接关系进行分析,来确定网页的重要性和相关性。
Python实现PageRank算法需要使用到矩阵运算和数学方法。下面是一个简单的Python实现PageRank算法的代码示例:
```
import numpy as np
def pageRank(G, s = .85, maxerr = .0001):
"""
Computes the pagerank for each of the n states
Parameters
----------
G: matrix representing state transitions
Gij is a binary value representing a transition from state i to j.
s: probability of following a transition. 1-s probability of teleporting
to another state. Defaults to 0.85
maxerr: if the sum of pageranks between iterations is bellow this we will
have converged. Defaults to 0.0001
"""
n = G.shape[0]
# transform G into markov matrix A
A = G / G.sum(axis=1)[:, np.newaxis]
# initialize pagerank r
r = np.ones(n) / n
# iterate till we converge
while True:
rnext = np.ones(n) * (1 - s) / n + s * A.T.dot(r)
diff = np.abs(rnext - r).sum()
r = rnext
if diff < maxerr:
return r
#example usage
G = np.array([[0,0,1],[0,1,1],[1,0,1]])
print(pageRank(G))
```
这个算法的输入是一个转移矩阵G,它表示网页之间的链接关系,每一行代表一个网页,每一列代表一个被链接到的网页。算法的输出是每个网页的PageRank值。
这只是一个简单的实现,实际应用中需要考虑更多的因素,比如网页之间的权重、网页的质量等。
阅读全文
相关推荐
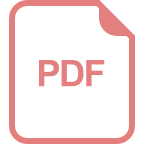

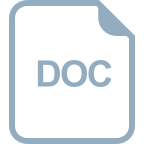

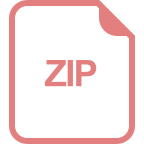
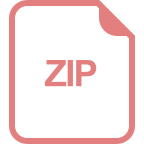
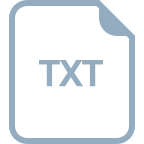
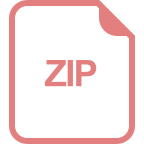




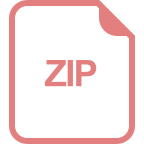