举个使用了strcmp的例子
时间: 2023-10-24 20:18:02 浏览: 36
假设我们有两个字符串str1和str2,我们想要比较它们是否相等,可以使用strcmp函数。
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[20] = "Hello";
char str2[20] = "Hello";
if (strcmp(str1, str2) == 0) {
printf("str1 and str2 are equal!\n");
} else {
printf("str1 and str2 are not equal!\n");
}
return 0;
}
```
在此示例中,我们首先包含了两个头文件,分别是stdio.h和string.h。然后,我们定义了两个字符串str1和str2,并将它们初始化为“Hello”。
接下来,我们使用strcmp函数比较这两个字符串。如果它们相等,strcmp函数将返回0,否则返回非零值。
最后,我们使用if语句检查strcmp函数的返回值。如果返回值为0,则输出“str1 and str2 are equal!”,否则输出“str1 and str2 are not equal!”。
相关问题
strcmp举个例子
strcmp是C语言中的字符串比较函数,用于比较两个字符串的大小。它的原型为:
```c
int strcmp(const char *str1, const char *str2);
```
其中,str1和str2是要比较的两个字符串。
strcmp函数的返回值有以下三种情况:
- 如果str1和str2相等,返回值为0;
- 如果str1大于str2,返回值大于0;
- 如果str1小于str2,返回值小于0。
下面是一个使用strcmp函数的例子:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "hello";
char str2[] = "world";
int result = strcmp(str1, str2);
if (result == 0) {
printf("str1和str2相等\n");
} else if (result > 0) {
printf("str1大于str2\n");
} else {
printf("str1小于str2\n");
}
return 0;
}
```
输出结果为:"str1小于str2",因为在字典序中,"hello"小于"world"。
strncmp与strcmp
strcmp和strncmp都是用来比较字符串的,区别在于strncmp是比较指定长度字符串,而strcmp则是比较整个字符串。两者都是二进制安全的,且区分大小写。在使用时,如果需要比较指定长度的字符串,则应该使用strncmp函数,否则使用strcmp函数即可。
举个例子,如果有两个字符串str1和str2,需要比较它们的前5个字符是否相同,可以使用strncmp(str1, str2, 5)函数来实现。如果需要比较整个字符串是否相同,则可以使用strcmp(str1, str2)函数来实现。
相关推荐
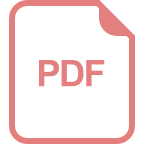












