std::thread 当成员变量时如何使用
时间: 2023-07-06 12:04:15 浏览: 75
当将 std::thread 当成员变量时,需要注意以下几点:
1. 在类的构造函数中初始化 std::thread 对象,可以使用成员初始化列表进行初始化。
2. 在类的析构函数中,需要判断线程是否已经加入或分离,如果没有则需要加入或分离线程,避免程序崩溃或出现未定义行为。
下面是一个示例代码:
```c++
#include <thread>
class MyClass {
public:
MyClass() : thread_(&MyClass::ThreadFunc, this) {}
~MyClass() {
if (thread_.joinable()) {
thread_.join();
}
}
private:
std::thread thread_;
void ThreadFunc() {
// 线程函数的实现
}
};
```
在上面的例子中,MyClass 类中有一个成员变量 thread_,它在构造函数中被初始化。在析构函数中,首先判断线程是否已经加入或分离,如果没有则调用 join() 方法加入线程。在 ThreadFunc() 方法中可以实现线程的具体逻辑。
相关问题
std::condition_variable和std::mutex可以不配合全局变量使用吗
std::condition_variable和std::mutex通常需要配合使用,以实现线程之间的同步和通信。std::mutex用于保护共享资源,而std::condition_variable用于在特定条件下等待和唤醒线程。
当一个线程需要等待某个条件满足时,它可以调用std::condition_variable的wait()函数,该函数会使线程进入等待状态,并释放对std::mutex的锁定。当其他线程满足了条件并调用了std::condition_variable的notify_one()或notify_all()函数时,等待的线程会被唤醒并重新获得对std::mutex的锁定,然后继续执行。
因此,std::condition_variable和std::mutex通常需要配合使用,以实现线程之间的同步和通信。如果不使用全局变量,那么线程之间无法共享条件变量和互斥锁,就无法实现线程之间的同步和通信。
示例代码如下所示:
```cpp
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
std::mutex g_mutex;
std::condition_variable g_cv;
bool g_flag = false;
void thread_func()
{
std::unique_lock<std::mutex> lock(g_mutex);
while (!g_flag)
{
g_cv.wait(lock);
}
std::cout << "Thread is awake!" << std::endl;
}
int main()
{
std::thread t(thread_func);
std::this_thread::sleep_for(std::chrono::seconds(2));
{
std::lock_guard<std::mutex> lock(g_mutex);
g_flag = true;
}
g_cv.notify_one();
t.join();
return 0;
}
```
将下列代码转换为Javausing namespace std; ADDRINT currentLocks [64]; // not all will be used and is to serve for programs with up to 64 worker threads. This is to catch real lock acquisitions std::unordered_map<ADDRINT, std::pair<vector<std::pair<THREADID, UINT32> >, bool> > memoryMap; std::unordered_map<ADDRINT, std::pair<vector<std::pair<THREADID, UINT32> >, bool> > readMemoryMap; std::unordered_map<ADDRINT, std::pair<vector<std::pair<THREADID, UINT32> >, bool> > lockMap; std::unordered_map<OS_THREAD_ID,THREADID> threadMap; std::unordered_map<THREADID, std::pair<UINT32, UINT32> > threadAbstract;
以下是将上述代码转换为 Java 代码的示例:
```java
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Vector;
public class PinTool {
private static ADDRINT[] currentLocks = new ADDRINT[64]; // not all will be used and is to serve for programs with up to 64 worker threads. This is to catch real lock acquisitions
private static Map<ADDRINT, Pair<List<Pair<THREADID, UINT32>>, Boolean>> memoryMap = new HashMap<>();
private static Map<ADDRINT, Pair<List<Pair<THREADID, UINT32>>, Boolean>> readMemoryMap = new HashMap<>();
private static Map<ADDRINT, Pair<List<Pair<THREADID, UINT32>>, Boolean>> lockMap = new HashMap<>();
private static Map<OS_THREAD_ID, THREADID> threadMap = new HashMap<>();
private static Map<THREADID, Pair<UINT32, UINT32>> threadAbstract = new HashMap<>();
public static void main(String[] args) {
// Your code here
}
private static class Pair<F, S> {
private F first;
private S second;
public Pair(F first, S second) {
this.first = first;
this.second = second;
}
public F getFirst() {
return first;
}
public S getSecond() {
return second;
}
public void setFirst(F first) {
this.first = first;
}
public void setSecond(S second) {
this.second = second;
}
}
private static class ThreadID {
// Your code here
}
}
```
请注意,Java 中没有直接等价于 C++ 中的 `unordered_map` 和 `vector` 的类。因此,我们使用了 Java 的 `HashMap` 和 `List` 来实现相似的功能。我们还定义了一个 `Pair` 类来存储键值对,类似于 C++ 中的 `std::pair`。
在以上示例中,我们定义了与 C++ 代码中的变量相对应的 Java 变量,并在 `main` 方法中留出了代码的位置供你添加其他逻辑。你可以根据具体需求修改和扩展这段代码,以满足你的需求。
请注意,由于无法完全转换 C++ 中的数据结构和语法到 Java 中,因此你可能需要根据实际情况进行适当的调整和修改。
阅读全文
相关推荐
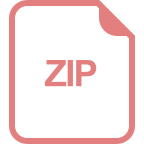
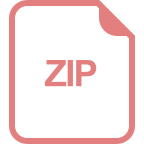
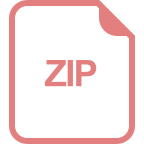
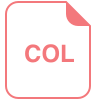












