使用c++l来读取execl当中的第十一行和第十二行数据,其中的数据格式为时间格式,将其按照时间中值排序
时间: 2024-02-19 07:58:29 浏览: 22
要读取Excel文件中的数据,可以使用 C++ 的第三方库,比如使用 `LibXL` 库。
以下是读取 Excel 文件中第 11 行和第 12 行数据的示例代码:
```c++
#include <iostream>
#include "libxl.h"
using namespace libxl;
int main() {
Book* book = xlCreateBook();
if (book) {
if (book->load("test.xlsx")) {
Sheet* sheet = book->getSheet(0);
if (sheet) {
// 读取第 11 行数据
int row = 10; // Excel 行数从 0 开始计数
int colCount = sheet->lastCol();
for (int i = 0; i < colCount; ++i) {
std::cout << sheet->readNum(row, i) << "\t";
}
std::cout << std::endl;
// 读取第 12 行数据
row = 11;
for (int i = 0; i < colCount; ++i) {
std::cout << sheet->readNum(row, i) << "\t";
}
std::cout << std::endl;
}
}
book->release();
}
return 0;
}
```
关于时间格式的排序,可以先将时间数据转换为时间戳(Unix 时间),然后按照时间戳排序。以下是一个简单的示例代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
// 将时间字符串转换为 Unix 时间戳
time_t toTimestamp(const std::string& str) {
struct tm t;
time_t timestamp;
strptime(str.c_str(), "%Y-%m-%d %H:%M:%S", &t);
timestamp = mktime(&t);
return timestamp;
}
int main() {
std::vector<std::string> data = {"2021-10-01 10:30:00", "2021-10-02 09:00:00", "2021-10-01 08:00:00", "2021-10-03 12:00:00"};
std::vector<time_t> timestamps;
for (const auto& str : data) {
timestamps.push_back(toTimestamp(str));
}
std::sort(timestamps.begin(), timestamps.end());
for (const auto& ts : timestamps) {
std::cout << std::ctime(&ts);
}
return 0;
}
```
该代码将时间字符串转换为 Unix 时间戳,并使用 `std::vector` 存储时间戳,然后使用 `std::sort` 函数对时间戳进行排序,并输出排序后的结果。
相关推荐
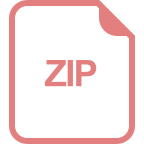














