我只想用python转换
时间: 2023-08-04 10:16:01 浏览: 46
如果您只想使用Python来转换Excel文件为E-R图,您可以使用以下步骤:
1. 使用Pandas库将Excel文件读入Python中,并将其转换为DataFrame对象。例如,您可以使用以下代码将Excel文件读入Python中:
```python
import pandas as pd
df = pd.read_excel('your_excel_file.xlsx')
```
2. 使用Pydot库创建一个空的E-R图,并创建所有的实体和关系。例如,您可以使用以下代码创建一个名为“mygraph”的空E-R图,并在其中创建名为“customer”和“order”的两个实体:
```python
import pydot
# Create an empty graph
graph = pydot.Dot(graph_type='graph', rankdir='LR')
# Create the customer entity
customer = pydot.Node('customer', shape='record', label='{<f0> customer_id|<f1> name|<f2> address}')
graph.add_node(customer)
# Create the order entity
order = pydot.Node('order', shape='record', label='{<f0> order_id|<f1> customer_id|<f2> order_date}')
graph.add_node(order)
# Create the relationship between customer and order
edge = pydot.Edge('customer:f0', 'order:f1', dir='none')
graph.add_edge(edge)
```
在这个示例中,我们创建了一个名为“customer”的实体,它有三个属性:customer_id、name和address。我们还创建了一个名为“order”的实体,它有三个属性:order_id、customer_id和order_date。最后,我们创建了一个连接“customer”的customer_id属性和“order”的customer_id属性的关系。
3. 将DataFrame对象中的数据添加到E-R图中。例如,您可以使用以下代码将DataFrame对象中的数据添加到“customer”实体和“order”实体中:
```python
# Loop through the rows of the DataFrame and add them to the graph
for index, row in df.iterrows():
# Create a new node for the customer
customer_node = pydot.Node('customer{}'.format(index), shape='record', label='{{<f0> {}|<f1> {}|<f2> {}}}'.format(row['customer_id'], row['name'], row['address']))
graph.add_node(customer_node)
# Create a new node for the order
order_node = pydot.Node('order{}'.format(index), shape='record', label='{{<f0> {}|<f1> {}|<f2> {}}}'.format(row['order_id'], row['customer_id'], row['order_date']))
graph.add_node(order_node)
# Create a new edge between the customer and the order
edge = pydot.Edge('customer{}:f0'.format(index), 'order{}:f1'.format(index), dir='none')
graph.add_edge(edge)
```
在这个示例中,我们循环遍历DataFrame对象中的每一行。为每行创建一个新的“customer”节点和一个新的“order”节点,并添加到E-R图中。我们还创建了一个新的关系,连接“customer”的customer_id属性和“order”的customer_id属性。
4. 使用Graph.write_png()方法将E-R图保存为PNG图像文件。例如,您可以使用以下代码将E-R图保存为“mygraph.png”文件:
```python
# Save the graph as a PNG image file
graph.write_png('mygraph.png')
```
这样,您就可以使用Python将Excel文件转换为E-R图了。
相关推荐
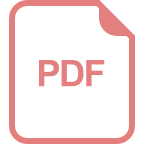
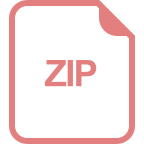
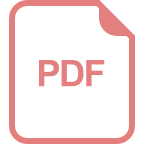














