读取ppm或pgm文件
时间: 2023-07-18 21:22:20 浏览: 182
好的,您可以使用以下代码读取ppm或pgm文件:
```python
def read_pgm_file(filename):
with open(filename, 'rb') as f:
# Read header
magic_number = f.readline()
if magic_number != b'P5\n' and magic_number != b'P2\n':
raise ValueError('Invalid magic number: {}'.format(magic_number))
comment = f.readline()
while comment.startswith(b'#'):
comment = f.readline()
width, height = map(int, comment.split())
max_val = int(f.readline().strip())
# Read image data
if magic_number == b'P5\n':
img = np.fromfile(f, dtype=np.uint8, count=width*height).reshape((height, width))
else:
img = np.zeros((height, width), dtype=np.uint8)
for i in range(height):
row = f.readline().split()
for j in range(width):
img[i, j] = int(row[j])
return img
def read_ppm_file(filename):
with open(filename, 'rb') as f:
# Read header
magic_number = f.readline()
if magic_number != b'P6\n' and magic_number != b'P3\n':
raise ValueError('Invalid magic number: {}'.format(magic_number))
comment = f.readline()
while comment.startswith(b'#'):
comment = f.readline()
width, height = map(int, comment.split())
max_val = int(f.readline().strip())
# Read image data
if magic_number == b'P6\n':
img = np.fromfile(f, dtype=np.uint8, count=3*width*height).reshape((height, width, 3))
else:
img = np.zeros((height, width, 3), dtype=np.uint8)
for i in range(height):
row = f.readline().split()
for j in range(width):
img[i, j, :] = [int(row[j*3+0]), int(row[j*3+1]), int(row[j*3+2])]
return img
```
这段代码使用numpy库以二进制格式读取ppm或pgm文件,并将其转换为numpy数组。其中,`read_pgm_file()`函数用于读取pgm文件,`read_ppm_file()`函数用于读取ppm文件。
阅读全文
相关推荐
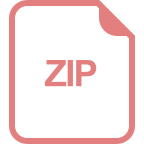
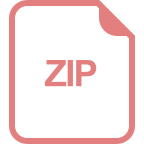
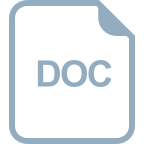











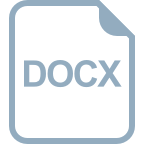