#define MAXSIZE 100 typedef int datatype; typedef struct { datatype a[MAXSIZE]; int size; } sequence_list; /* 函数功能:顺序表的初始化-置空表 */ void init(sequence_list *slt); /* 函数功能:在顺序表后部进行插入操作 */ void append(sequence_list *slt, datatype x); /* 函数功能:打印顺序表的各结点值 */ void display(sequence_list slt); /* 函数功能:判断顺序表是否为空 */ int empty(sequence_list slt); /* 函数功能:查找顺序表中值为x的结点位置 */ int find(sequence_list slt, datatype x); /* 函数功能:取得顺序表中第i个结点的值 */ datatype get(sequence_list slt, int i); /* 函数功能:在顺序表的position位置插入值为x的结点 */ void insert(sequence_list *slt, datatype x, int position); /* 函数功能:删除顺序表中第position位置的结点 */ void dele(sequence_list *slt, int position); 为这段代码编写测试用例
时间: 2024-03-22 17:42:38 浏览: 22
测试用例如下:
```c
#include <stdio.h>
#include "sequence_list.h"
int main() {
sequence_list slt;
int i;
// 初始化
init(&slt);
printf("初始化后,顺序表是否为空:%d\n", empty(slt));
// 插入结点
for (i = 1; i <= 5; i++) {
append(&slt, i);
}
printf("插入5个结点后,顺序表是否为空:%d\n", empty(slt));
printf("顺序表中各结点值为:");
display(slt);
// 查找结点
printf("值为3的结点位置:%d\n", find(slt, 3));
printf("值为7的结点位置:%d\n", find(slt, 7));
// 获取结点
printf("第3个结点的值:%d\n", get(slt, 3));
// 插入结点
insert(&slt, 6, 3);
printf("在第3个位置插入值为6的结点后,顺序表中各结点值为:");
display(slt);
// 删除结点
dele(&slt, 5);
printf("删除第5个结点后,顺序表中各结点值为:");
display(slt);
return 0;
}
```
相关推荐
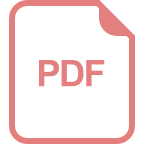
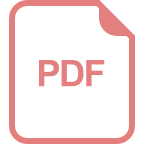
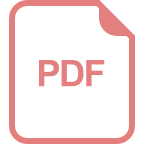















