#include "main.h" #include "usart.h" #include "dht11.h" #include "delay.h" #include "BC20.h" #include <string.h> extern char cardid[40]; int main(void) { u8 temp = 0, humi = 0; char len[20] = {0},data[50] = {0}; int ret = 0; delay_init(); uart1_init(115200); uart2_init(115200); uart3_init(115200); while(DHT11_Init()); printf("=======DHT11 init complete=======\n"); while(BC20_Init()){}; BC20_PDPACT(); BC20_ConUDP(); printf("=======BC20 init complete=======\n"); while(1){ DHT11_Read_Data(&temp,&humi); printf("card_id is %s, temp = %d,humi = %d\n",cardid,temp,humi); ret = sprintf(data, "%s+temp = %d, humi = %d",cardid,temp,humi); sprintf(len,"%d",ret); BC20_Senddata((uint8_t *)len,(uint8_t *)data); Delay(1000); BC20_RECData(); delay_ms(2000); } }解释这段代码
时间: 2024-01-23 16:04:01 浏览: 280
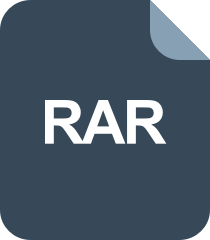
USART.rar_stm32f10x_it.h
这段代码是一个嵌入式系统的主函数,主要实现了读取DHT11温湿度传感器的数据,发送数据到SIM7600CE模块(也称为BC20),并通过串口打印调试信息。
具体来说,代码中包含了以下头文件:
```c
#include "main.h"
#include "usart.h"
#include "dht11.h"
#include "delay.h"
#include "BC20.h"
#include <string.h>
```
其中,`main.h`和`usart.h`是系统自带的头文件,`dht11.h`是DHT11温湿度传感器驱动程序的头文件,`delay.h`是延时函数的头文件,`BC20.h`是SIM7600CE模块的驱动程序头文件,`string.h`是C语言标准库中的字符串操作函数头文件。
接着,代码定义了一个全局变量`cardid`,类型为字符数组,长度为40:
```c
extern char cardid[40];
```
该变量用于保存设备的ID号。
在`main`函数中,代码初始化了延时函数和三个串口:
```c
delay_init();
uart1_init(115200);
uart2_init(115200);
uart3_init(115200);
```
然后,代码使用`DHT11_Init`函数来初始化DHT11温湿度传感器,使用`BC20_Init`函数来初始化SIM7600CE模块,使用`BC20_PDPACT`函数来激活PDP上下文,使用`BC20_ConUDP`函数来建立UDP连接:
```c
while(DHT11_Init());
printf("=======DHT11 init complete=======\n");
while(BC20_Init()){};
BC20_PDPACT();
BC20_ConUDP();
printf("=======BC20 init complete=======\n");
```
接着,代码进入一个无限循环,在每次循环中使用`DHT11_Read_Data`函数读取DHT11传感器的温湿度数据,使用`sprintf`函数将数据转换为字符串并保存在`data`数组中,然后使用`sprintf`函数将字符串的长度保存在`len`数组中,最后使用`BC20_Senddata`函数将数据发送到远程服务器:
```c
while(1){
DHT11_Read_Data(&temp,&humi);
printf("card_id is %s, temp = %d,humi = %d\n",cardid,temp,humi);
ret = sprintf(data, "%s+temp = %d, humi = %d",cardid,temp,humi);
sprintf(len,"%d",ret);
BC20_Senddata((uint8_t *)len,(uint8_t *)data);
Delay(1000);
BC20_RECData();
delay_ms(2000);
}
```
同时,代码还使用`printf`函数将调试信息输出到串口。
总体来说,这段代码实现了一个简单的温湿度数据采集和发送的功能,适用于嵌入式系统中对温湿度数据进行实时监测的场景。
阅读全文
相关推荐
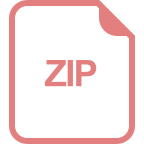
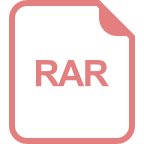















