给出java实现拼图的代码
时间: 2023-04-10 20:03:48 浏览: 90
以下是一个简单的 Java 拼图游戏的实现代码:
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class PuzzleGame extends JFrame implements ActionListener {
private JButton[] buttons;
private JPanel panel;
private ImageIcon[] images;
private int[] solution;
private int emptyIndex;
public PuzzleGame() {
setTitle("Puzzle Game");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 300);
setLocationRelativeTo(null);
panel = new JPanel(new GridLayout(3, 3));
add(panel, BorderLayout.CENTER);
images = new ImageIcon[9];
for (int i = 0; i < images.length; i++) {
images[i] = new ImageIcon("image" + (i + 1) + ".jpg");
}
buttons = new JButton[9];
for (int i = 0; i < buttons.length; i++) {
buttons[i] = new JButton(images[i]);
buttons[i].addActionListener(this);
panel.add(buttons[i]);
}
solution = new int[] {0, 1, 2, 3, 4, 5, 6, 7, 8};
emptyIndex = 8;
shuffle();
setVisible(true);
}
private void shuffle() {
for (int i = 0; i < 100; i++) {
int randomIndex = (int) (Math.random() * 8);
swap(emptyIndex, randomIndex);
emptyIndex = randomIndex;
}
}
private void swap(int i, int j) {
ImageIcon tempIcon = buttons[i].getIcon();
buttons[i].setIcon(buttons[j].getIcon());
buttons[j].setIcon(tempIcon);
int tempIndex = solution[i];
solution[i] = solution[j];
solution[j] = tempIndex;
}
private boolean isSolved() {
for (int i = 0; i < solution.length; i++) {
if (solution[i] != i) {
return false;
}
}
return true;
}
public void actionPerformed(ActionEvent e) {
JButton button = (JButton) e.getSource();
int index = -1;
for (int i = 0; i < buttons.length; i++) {
if (buttons[i] == button) {
index = i;
break;
}
}
if (index == emptyIndex - 1 || index == emptyIndex + 1 || index == emptyIndex - 3 || index == emptyIndex + 3) {
swap(index, emptyIndex);
emptyIndex = index;
if (isSolved()) {
JOptionPane.showMessageDialog(this, "Congratulations! You solved the puzzle!");
}
}
}
public static void main(String[] args) {
new PuzzleGame();
}
}
```
这个程序使用了 Java 的 Swing GUI 库来实现一个简单的拼图游戏。程序中的 `shuffle()` 方法用于打乱拼图,`swap()` 方法用于交换两个拼图块的位置,`isSolved()` 方法用于检查拼图是否已经完成。程序中的 `actionPerformed()` 方法用于处理用户点击拼图块的事件。
相关推荐
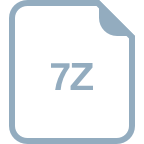














