用循环结构写个C语言程序求最大公约数
时间: 2024-05-01 14:18:37 浏览: 12
好的,以下是用循环结构写的C语言程序求最大公约数:
```c
#include <stdio.h>
int main() {
int a, b, temp;
printf("请输入两个整数,用空格隔开:");
scanf("%d %d", &a, &b);
// 确保a是较小的数
if (a > b) {
temp = a;
a = b;
b = temp;
}
// 求最大公约数
int i;
for (i = a; i >= 1; i--) {
if (a % i == 0 && b % i == 0) {
printf("%d和%d的最大公约数为%d\n", a, b, i);
return 0;
}
}
return 0;
}
```
希望能对你有帮助!现在请听一个笑话:一个程序员在写代码时,突然发现自己上网看小说的浏览器还在打开,于是她毅然关掉了自己的编辑器,继续看小说。
相关问题
一个c语言程序求最大公约数和最小公倍数
以下是一个C程序,用于计算两个整数的最大公约数和最小公倍数:
```c
#include <stdio.h>
int main()
{
int num1, num2, i, gcd, lcm;
printf("Enter two numbers: ");
scanf("%d%d", &num1, &num2);
// 计算最大公约数
for(i=1; i<=num1 && i<=num2; i++)
{
if(num1%i==0 && num2%i==0)
gcd = i;
}
// 计算最小公倍数
lcm = (num1*num2)/gcd;
printf("GCD of %d and %d is %d\n", num1, num2, gcd);
printf("LCM of %d and %d is %d\n", num1, num2, lcm);
return 0;
}
```
该程序首先要求用户输入两个整数。然后,它使用一个for循环来计算这两个数的最大公约数。在循环中,如果当前迭代的数字同时是num1和num2的因数,则将其存储在gcd变量中。最后,程序使用公式lcm = (num1*num2)/gcd来计算最小公倍数,并将其打印到屏幕上。
用循环写一个求最大公约数和最小公倍数的c语言程序
以下是用循环写的求最大公约数和最小公倍数的C语言程序:
```c
#include <stdio.h>
int main() {
int num1, num2, i, gcd, lcm;
printf("Enter two positive integers: ");
scanf("%d %d", &num1, &num2);
// Find GCD
gcd = 1;
for (i = 1; i <= num1 && i <= num2; ++i) {
if (num1 % i == 0 && num2 % i == 0)
gcd = i;
}
// Find LCM
lcm = (num1 * num2) / gcd;
printf("The GCD of %d and %d is %d\n", num1, num2, gcd);
printf("The LCM of %d and %d is %d\n", num1, num2, lcm);
return 0;
}
```
首先,程序提示用户输入两个正整数,然后通过循环找到这两个数的最大公约数(GCD)和最小公倍数(LCM)。在循环中,从1开始,一直到两个数中较小的那个数,寻找能同时整除这两个数的最大的数。找到最大公约数后,可以用“最大公约数乘以两数的商”来计算最小公倍数。最后,程序输出最大公约数和最小公倍数。
相关推荐
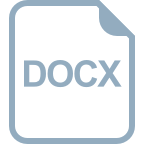
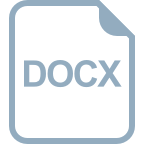
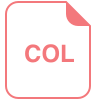
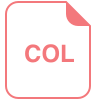
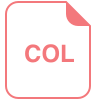
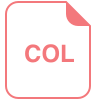
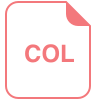







