写一个这样的代码void insert_item(char *, float, float) -add a new item and its price into linked list
时间: 2024-02-20 20:59:36 浏览: 20
Sure, here's an example implementation of the `insert_item` function that adds a new item and its price into a linked list:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Node {
char *name;
float price;
float amount_paid;
struct Node *next;
} Node;
Node *head = NULL;
void insert_item(char *name, float price, float amount_paid) {
Node *new_node = (Node*) malloc(sizeof(Node));
new_node->name = strdup(name);
new_node->price = price;
new_node->amount_paid = amount_paid;
new_node->next = NULL;
if (head == NULL) {
head = new_node;
} else {
Node *current = head;
Node *prev = NULL;
while (current != NULL && current->price > price) {
prev = current;
current = current->next;
}
if (prev == NULL) {
new_node->next = head;
head = new_node;
} else {
prev->next = new_node;
new_node->next = current;
}
}
}
```
This function creates a new node with the provided name, price, and amount_paid values, and then inserts it into the linked list in descending order based on the price. If the list is empty, the new node is set as the head. If not, the function iterates through the list until it finds the correct position to insert the new node, and adjusts the pointers accordingly.
Note that this implementation assumes that the linked list is already defined and initialized elsewhere in the program.
相关推荐
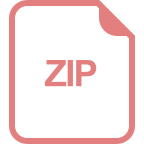
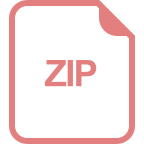














