draw = ImageDraw.Draw(im)什么意思
时间: 2023-06-07 19:11:42 浏览: 69
这段代码是在Python中使用PIL库对一张图片对象进行编辑,其中draw是一个用于绘图的对象,ImageDraw是PIL库中的一个绘图库,而im则是代表了一张图片。这段代码的作用是创建一个用于在图片上进行绘图的对象。
相关问题
im.load() if im.readonly: im._copy() # make it writeable blend = 0 if mode is None: mode = im.mode if mode != im.mode: if mode == "RGBA" and im.mode == "RGB": blend = 1 else: msg = "mode mismatch" raise ValueError(msg) if mode == "P": self.palette = im.palette else: self.palette = None self._image = im self.im = im.im self.draw = Image.core.draw(self.im, blend) self.mode = mode if mode in ("I", "F"): self.ink = self.draw.draw_ink(1) else: self.ink = self.draw.draw_ink(-1) if mode in ("1", "P", "I", "F"): # FIXME: fix Fill2 to properly support matte for I+F images self.fontmode = "1" else: self.fontmode = "L" # aliasing is okay for other modes self.fill = False西边是这段代码的错误,应该怎么修改 File "F:\ana\anaconda3\envs\torch\lib\site-packages\PIL\ImageDraw.py", line 62, in __init__ im.load() AttributeError: 'numpy.ndarray' object has no attribute 'load'
根据代码的错误提示,'numpy.ndarray'对象没有'load'属性,所以你需要将im对象更改为一个PIL图像对象,而不是一个numpy数组对象。
你可以使用PIL库中的`Image.fromarray()`方法将numpy数组转换为PIL图像对象,然后再进行加载和操作。修改后的代码如下所示:
```python
from PIL import Image
# 将numpy数组转换为PIL图像对象
im = Image.fromarray(im)
im.load()
if im.readonly:
im._copy() # make it writeable
blend = 0
if mode is None:
mode = im.mode
if mode != im.mode:
if mode == "RGBA" and im.mode == "RGB":
blend = 1
else:
msg = "mode mismatch"
raise ValueError(msg)
if mode == "P":
self.palette = im.palette
else:
self.palette = None
self._image = im
self.im = im.im
self.draw = Image.core.draw(self.im, blend)
self.mode = mode
if mode in ("I", "F"):
self.ink = self.draw.draw_ink(1)
else:
self.ink = self.draw.draw_ink(-1)
if mode in ("1", "P", "I", "F"):
# FIXME: fix Fill2 to properly support matte for I+F images
self.fontmode = "1"
else:
self.fontmode = "L" # aliasing is okay for other modes
self.fill = False
```
这样,你就可以在加载numpy数组之前将其转换为PIL图像对象,然后在代码中继续使用它。
from PIL import Image, ImageDraw, ImageFont import os text = "watermark" # 水印文字 font = ImageFont.truetype("arial.ttf", 36) # 字体和大小 path = r"C:\Users\Administrator\Desktop\0424特价直播样品\新建文件夹\新建文件夹" for filename in os.listdir(path): print(path,filename) if filename.endswith(".jpg") or filename.endswith(".png") or filename.endswith(".JPG") or filename.endswith(".PNG"): # 只处理jpg和png图片 # 处理每一张图片 # 打开图片 image = Image.open(os.path.join(path, filename)) print(image) # 新建一个图层,用于绘制水印 layer = Image.new("RGBA", image.size, (0,0,0,0)) draw = ImageDraw.Draw(layer) # 计算水印文字的位置 text_width, text_height = draw.textsize(text, font) x = image.width - text_width - 10 # x坐标 y = image.height - text_height - 10 # y坐标 # 绘制水印文字 draw.text((x,y), text, font=font, fill=(255,255,255,128)) # 合并图层 result = Image.alpha_composite(image, layer) print(result) # 保存处理后的图片 result.save(os.path.join(path, filename)) 优化这段代码 当前报错内容 File "E:\python-i\venv\lib\site-packages\PIL\Image.py", line 3168, in alpha_composite return im1._new(core.alpha_composite(im1.im, im2.im)) ValueError: image has wrong mode 图片是mode=RGB
根据你提供的代码和报错信息,可能是因为调用了 `alpha_composite()` 方法,该方法要求两张图片的模式必须相同。因此,你需要将新建的图层 `layer` 和打开的图片 `image` 都转换为 RGBA 模式才可以使用 `alpha_composite()` 方法。
以下是修改后的代码:
```python
from PIL import Image, ImageDraw, ImageFont
import os
text = "watermark" # 水印文字
font = ImageFont.truetype("arial.ttf", 36) # 字体和大小
path = r"C:\Users\Administrator\Desktop\0424特价直播样品\新建文件夹\新建文件夹"
for filename in os.listdir(path):
if filename.endswith(".jpg") or filename.endswith(".png") or filename.endswith(".JPG") or filename.endswith(".PNG"): # 只处理jpg和png图片
# 处理每一张图片
# 打开图片
image = Image.open(os.path.join(path, filename))
print(image.mode)
# 将图片转换为 RGBA 模式
if image.mode != 'RGBA':
image = image.convert('RGBA')
# 新建一个图层,用于绘制水印
layer = Image.new("RGBA", image.size, (0,0,0,0))
draw = ImageDraw.Draw(layer)
# 计算水印文字的位置
text_width, text_height = draw.textsize(text, font)
x = image.width - text_width - 10 # x坐标
y = image.height - text_height - 10 # y坐标
# 绘制水印文字
draw.text((x,y), text, font=font, fill=(255,255,255,128))
# 将图层转换为 RGBA 模式
if layer.mode != 'RGBA':
layer = layer.convert('RGBA')
# 合并图层
result = Image.alpha_composite(image, layer)
print(result.mode)
# 保存处理后的图片
result.save(os.path.join(path, filename))
```
在上述代码中,我们添加了两个 `if` 语句,用于检查图片和图层的模式是否为 RGBA 模式。如果不是,则使用 `convert()` 方法将其转换为 RGBA 模式。这样就可以避免 `alpha_composite()` 方法报错的问题。
相关推荐
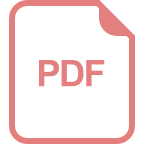
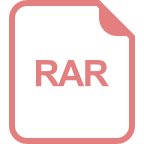












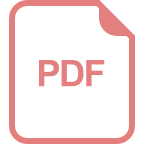