一个班有N名学生,每个学生修了五门课。 1)求每个学生的平均成绩,并输出每个学生的学号,每门课程的成绩及平均值。 2)求某门课程的平均分; 1、分别编写2个函数实现以上2个要求。 2、第1个函数用数组名作参数。第2个函数用指针作参数,并在函数体内用指针对数组操作。 输入
时间: 2024-02-24 15:53:44 浏览: 34
好的,下面是一个 C 语言程序,使用了数组名和指针分别实现了以上两个要求:
```c
#include <stdio.h>
#define MAX_STUDENT 50
#define COURSE_NUM 5
void print_student_info(int scores[][COURSE_NUM], int n) {
int i, j;
float avg;
printf("学号\t课程1\t课程2\t课程3\t课程4\t课程5\t平均分\n");
for (i = 0; i < n; i++) {
printf("%d\t", i + 1);
avg = 0;
for (j = 0; j < COURSE_NUM; j++) {
printf("%d\t", scores[i][j]);
avg += scores[i][j];
}
avg /= COURSE_NUM;
printf("%.2f\n", avg);
}
}
void print_course_avg(int (*scores)[COURSE_NUM], int n, int course) {
int i;
float avg = 0;
for (i = 0; i < n; i++) {
avg += scores[i][course - 1];
}
avg /= n;
printf("课程%d的平均分为:%.2f\n", course, avg);
}
int main() {
int scores[MAX_STUDENT][COURSE_NUM];
int i, j, course;
// 输入学生成绩
printf("请输入学生的成绩:\n");
for (i = 0; i < MAX_STUDENT; i++) {
printf("请输入第 %d 个学生的成绩:\n", i + 1);
for (j = 0; j < COURSE_NUM; j++) {
printf("请输入第 %d 门课的成绩:", j + 1);
scanf("%d", &scores[i][j]);
}
}
// 输出每个学生的平均成绩及课程成绩
printf("每个学生的平均成绩及课程成绩如下:\n");
print_student_info(scores, MAX_STUDENT);
// 输出某门课程的平均分
printf("请输入要求平均分的课程编号(1-5):");
scanf("%d", &course);
print_course_avg(scores, MAX_STUDENT, course);
return 0;
}
```
该程序中,`print_student_info` 函数用于输出每个学生的平均成绩及课程成绩,它使用了数组名作为参数。`print_course_avg` 函数用于输出某门课程的平均分,它使用了指针作为参数,并在函数体内用指针对数组进行操作。在 `main` 函数中,先输入学生成绩,然后分别调用这两个函数输出结果。
相关推荐
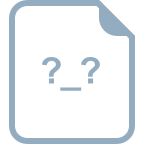
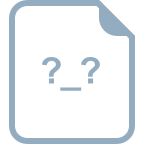















