基于小波变换的特征提取法 代码
时间: 2024-03-22 18:37:51 浏览: 13
基于小波变换的特征提取法具体实现可以使用以下步骤:
1. 加载音频文件,并将其转换为时域信号。
```Python
import librosa
import numpy as np
# 读取音频文件
y, sr = librosa.load('audio_file.wav', sr=None)
```
2. 对时域信号进行小波变换。
```Python
import pywt
# 小波变换
coeffs = pywt.wavedec(y, 'db4', level=4)
```
3. 从小波系数中提取特征。
```Python
# 特征提取
features = []
for i, c in enumerate(coeffs):
c_mean = np.mean(c)
c_std = np.std(c)
c_var = np.var(c)
c_energy = np.sum(np.square(c))
c_entropy = -np.sum(np.square(c) * np.log2(np.square(c) + 1e-6))
features.extend([c_mean, c_std, c_var, c_energy, c_entropy])
```
4. 对特征进行归一化处理。
```Python
from sklearn.preprocessing import StandardScaler
# 特征归一化
scaler = StandardScaler()
features_norm = scaler.fit_transform(np.array(features).reshape(1, -1))
```
完整代码:
```Python
import librosa
import numpy as np
import pywt
from sklearn.preprocessing import StandardScaler
# 读取音频文件
y, sr = librosa.load('audio_file.wav', sr=None)
# 小波变换
coeffs = pywt.wavedec(y, 'db4', level=4)
# 特征提取
features = []
for i, c in enumerate(coeffs):
c_mean = np.mean(c)
c_std = np.std(c)
c_var = np.var(c)
c_energy = np.sum(np.square(c))
c_entropy = -np.sum(np.square(c) * np.log2(np.square(c) + 1e-6))
features.extend([c_mean, c_std, c_var, c_energy, c_entropy])
# 特征归一化
scaler = StandardScaler()
features_norm = scaler.fit_transform(np.array(features).reshape(1, -1))
```
相关推荐
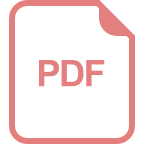
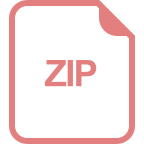
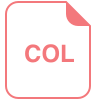
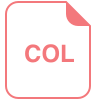
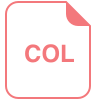
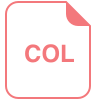
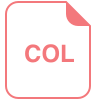









