利用编程从Hbase读取学生成绩,并实现每个学生成绩平均分和每门课程成绩平均分
时间: 2024-02-04 14:02:07 浏览: 74
好的,这是一个比较复杂的问题,需要分步骤来实现。首先,需要连接到 HBase 数据库并读取学生成绩数据。这可以通过以下代码实现:
```python
import happybase
# 连接到 HBase 数据库
connection = happybase.Connection(host='localhost', port=9090)
# 选择一个表
table = connection.table('student_scores')
# 读取所有行数据
rows = table.scan()
# 打印所有行数据
for key, data in rows:
print(key, data)
```
接下来,需要解析每个学生的成绩数据,并计算每个学生的平均分和每门课程的平均分。这可以通过以下代码实现:
```python
# 创建一个字典来存储每个学生的成绩数据
student_scores = {}
# 遍历所有行数据
for key, data in rows:
# 解析行数据
student_id = key.decode('utf-8')
subject = data[b'subject'].decode('utf-8')
score = int(data[b'score'].decode('utf-8'))
# 将成绩数据添加到字典中
if student_id not in student_scores:
student_scores[student_id] = {}
if subject not in student_scores[student_id]:
student_scores[student_id][subject] = []
student_scores[student_id][subject].append(score)
# 计算每个学生的平均分和每门课程的平均分
for student_id in student_scores:
# 计算每个学生的平均分
total_score = 0
total_subjects = 0
for subject in student_scores[student_id]:
total_score += sum(student_scores[student_id][subject])
total_subjects += len(student_scores[student_id][subject])
average_score = total_score / total_subjects
print(f'Student {student_id} average score: {average_score}')
# 计算每门课程的平均分
for subject in student_scores[student_id]:
average_subject_score = sum(student_scores[student_id][subject]) / len(student_scores[student_id][subject])
print(f'Subject {subject} average score: {average_subject_score}')
```
这段代码将所有成绩数据存储在一个字典中,并计算每个学生的平均分和每门课程的平均分。注意,在计算每个学生的平均分时,需要计算所有科目的总分数和总科目数,而不是只计算每个科目的平均分数。
阅读全文
相关推荐
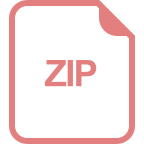
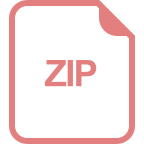
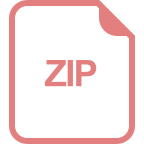








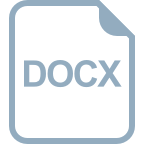
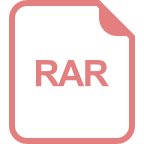
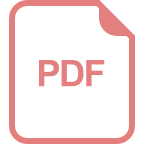
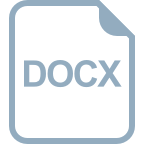
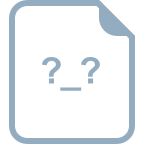
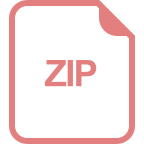
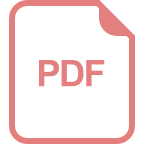