编程,输入 n(3<n<=10)个职工的编号、姓名、基本工资、职务工资,输 出其中“基本工资+职务工资”最少和最多的职工姓名。 输入输出示例: n=5 1,林宏,1800,600 2,李涛,1700,550 3,王钢,2100,750 4,周航,1500,450 5,金明,2300,850 基本工资+职务工资最不的是:周航 基本工资+职务工资最多的是:金明 6.编程,建立一个有 n(3<n<=10)个学生成绩的结构记录,包括学号、姓名 和 3 门成绩,输出总分最高学生的姓名和总分。用c语言
时间: 2024-02-09 08:12:34 浏览: 22
以下是第一题的示例代码:
```c
#include <stdio.h>
#include <string.h>
struct Employee {
int id;
char name[20];
int basicSalary;
int positionSalary;
};
int main() {
int n, minIndex, maxIndex, minSalary = 0x7fffffff, maxSalary = -0x7fffffff;
struct Employee employees[10];
printf("Enter the number of employees (3<=n<=10): ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("Enter employee #%d's id, name, basicSalary and positionSalary: ", i+1);
scanf("%d %s %d %d", &employees[i].id, employees[i].name, &employees[i].basicSalary, &employees[i].positionSalary);
int totalSalary = employees[i].basicSalary + employees[i].positionSalary;
if (totalSalary < minSalary) {
minSalary = totalSalary;
minIndex = i;
}
if (totalSalary > maxSalary) {
maxSalary = totalSalary;
maxIndex = i;
}
}
printf("The employee with the lowest total salary is: %s\n", employees[minIndex].name);
printf("The employee with the highest total salary is: %s\n", employees[maxIndex].name);
return 0;
}
```
输入职工的信息,然后使用循环计算出每个职工的总工资,同时记录下最少和最多的职工的索引。最后输出这两个职工的姓名。
以下是第二题的示例代码:
```c
#include <stdio.h>
#include <string.h>
struct Student {
int id;
char name[20];
int score1;
int score2;
int score3;
};
int main() {
int n, maxIndex, maxScore = -0x7fffffff;
struct Student students[10];
printf("Enter the number of students (3<=n<=10): ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("Enter student #%d's id, name, score1, score2 and score3: ", i+1);
scanf("%d %s %d %d %d", &students[i].id, students[i].name, &students[i].score1, &students[i].score2, &students[i].score3);
int totalScore = students[i].score1 + students[i].score2 + students[i].score3;
if (totalScore > maxScore) {
maxScore = totalScore;
maxIndex = i;
}
}
printf("The student with the highest total score is: %s\n", students[maxIndex].name);
printf("The total score is: %d\n", maxScore);
return 0;
}
```
输入学生的信息,然后使用循环计算出每个学生的总分数,同时记录下总分最高的学生的索引。最后输出这个学生的姓名和总分数。
相关推荐
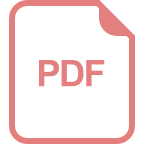














