c++ 读取 hdf5文件,文件里包括info和data两种数据。info为字符串字典,data为两维数据
时间: 2024-02-18 14:02:38 浏览: 145
你可以使用HDF5 C++ API来读取HDF5文件中的数据。下面是一个简单的示例代码,可以读取HDF5文件中名为"info"和"data"的两个数据集:
```c++
#include <iostream>
#include <string>
#include "H5Cpp.h"
const std::string FILE_NAME("your_file_name.h5");
const std::string INFO_DATASET_NAME("info");
const std::string DATA_DATASET_NAME("data");
int main()
{
// Open the HDF5 file
H5::H5File file(FILE_NAME, H5F_ACC_RDONLY);
// Read the "info" dataset
H5::DataSet info_dataset = file.openDataSet(INFO_DATASET_NAME);
H5::DataSpace info_dataspace = info_dataset.getSpace();
// Get the number of elements in the dataset
int info_size = info_dataspace.getSimpleExtentNpoints();
// Allocate memory to hold the data
char* info_buf = new char[info_size+1];
// Read the data from the dataset into the memory buffer
info_dataset.read(info_buf, H5::PredType::NATIVE_CHAR);
// Null-terminate the string
info_buf[info_size] = '\0';
// Print the info string
std::cout << "info: " << info_buf << std::endl;
// Clean up the memory
delete[] info_buf;
// Read the "data" dataset
H5::DataSet data_dataset = file.openDataSet(DATA_DATASET_NAME);
H5::DataSpace data_dataspace = data_dataset.getSpace();
// Get the dimensions of the dataset
int ndims = data_dataspace.getSimpleExtentNdims();
hsize_t dims[ndims];
data_dataspace.getSimpleExtentDims(dims);
// Allocate memory to hold the data
double* data_buf = new double[dims[0]*dims[1]];
// Define the hyperslab that we want to read
hsize_t offset[2] = {0, 0};
hsize_t count[2] = {dims[0], dims[1]};
data_dataspace.selectHyperslab(H5S_SELECT_SET, count, offset);
// Define the memory dataspace
H5::DataSpace mem_dataspace(ndims, dims);
// Read the data from the dataset into the memory buffer
data_dataset.read(data_buf, H5::PredType::NATIVE_DOUBLE, mem_dataspace, data_dataspace);
// Print the data
std::cout << "data:" << std::endl;
for (int i = 0; i < dims[0]; i++) {
for (int j = 0; j < dims[1]; j++) {
std::cout << data_buf[i*dims[1]+j] << " ";
}
std::cout << std::endl;
}
// Clean up the memory
delete[] data_buf;
return 0;
}
```
上述代码使用了H5Cpp.h头文件中定义的C++类来读取HDF5文件中的数据。首先,我们打开文件并读取"info"和"data"数据集。对于"info"数据集,我们直接将数据读取到一个char数组中,然后用cout输出即可。对于"data"数据集,我们需要手动定义一个内存缓冲区,并使用selectHyperslab方法来指定需要读取的数据范围。最后,我们将数据读取到内存缓冲区中,并输出到控制台。
阅读全文
相关推荐
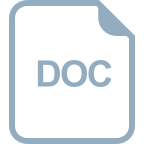
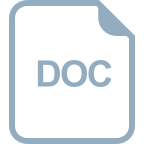
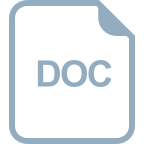
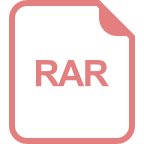
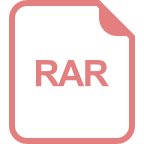












