我想在以下这段代码中,添加显示标有特征点的图像的功能。def cnn_feature_extract(image,scales=[.25, 0.50, 1.0], nfeatures = 1000): if len(image.shape) == 2: image = image[:, :, np.newaxis] image = np.repeat(image, 3, -1) # TODO: switch to PIL.Image due to deprecation of scipy.misc.imresize. resized_image = image if max(resized_image.shape) > max_edge: resized_image = scipy.misc.imresize( resized_image, max_edge / max(resized_image.shape) ).astype('float') if sum(resized_image.shape[: 2]) > max_sum_edges: resized_image = scipy.misc.imresize( resized_image, max_sum_edges / sum(resized_image.shape[: 2]) ).astype('float') fact_i = image.shape[0] / resized_image.shape[0] fact_j = image.shape[1] / resized_image.shape[1] input_image = preprocess_image( resized_image, preprocessing="torch" ) with torch.no_grad(): if multiscale: keypoints, scores, descriptors = process_multiscale( torch.tensor( input_image[np.newaxis, :, :, :].astype(np.float32), device=device ), model, scales ) else: keypoints, scores, descriptors = process_multiscale( torch.tensor( input_image[np.newaxis, :, :, :].astype(np.float32), device=device ), model, scales ) # Input image coordinates keypoints[:, 0] *= fact_i keypoints[:, 1] *= fact_j # i, j -> u, v keypoints = keypoints[:, [1, 0, 2]] if nfeatures != -1: #根据scores排序 scores2 = np.array([scores]).T res = np.hstack((scores2, keypoints)) res = res[np.lexsort(-res[:, ::-1].T)] res = np.hstack((res, descriptors)) #取前几个 scores = res[0:nfeatures, 0].copy() keypoints = res[0:nfeatures, 1:4].copy() descriptors = res[0:nfeatures, 4:].copy() del res return keypoints, scores, descriptors
时间: 2024-01-04 20:02:04 浏览: 131
可以使用OpenCV库中的cv2.drawKeypoints()函数来显示标有特征点的图像。具体实现如下:
1. 导入OpenCV库:import cv2
2. 在函数中添加以下代码,绘制特征点:
```
img_with_keypoints = cv2.drawKeypoints(image, keypoints, np.array([]), (255,0,0), cv2.DRAW_MATCHES_FLAGS_DRAW_RICH_KEYPOINTS)
cv2.imshow("Image with Keypoints", img_with_keypoints)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码将在窗口中显示标有特征点的图像。注意,要在函数中添加完整的代码,包括导入OpenCV库等。
阅读全文
相关推荐
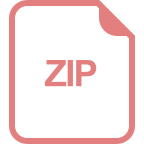
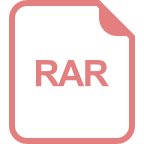
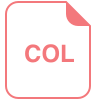
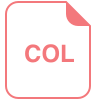
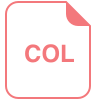
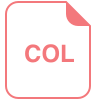
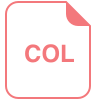
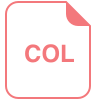
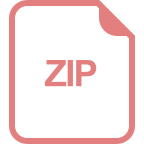
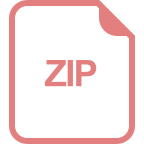