现有的目标检测有哪些好用的模型,并分别用python举例说明
时间: 2023-06-10 08:05:37 浏览: 89
目前在目标检测领域,有不少好用的模型,以下是其中几个比较有名且效果比较好的模型,以及用Python实现的代码举例:
1. Faster R-CNN:Faster R-CNN是一种基于Region Proposal Network(RPN)的目标检测算法,它比较适合用于对小物体的检测。Python实现代码如下:
```python
import torch
import torchvision
from torchvision.models.detection.faster_rcnn import FastRCNNPredictor
# 加载预训练的 Faster R-CNN 模型
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
# 用 Faster R-CNN 模型中的分类器替换掉预训练模型中的分类器
num_classes = 2 # 假设我们只有两种物体需要检测
in_features = model.roi_heads.box_predictor.cls_score.in_features
model.roi_heads.box_predictor = FastRCNNPredictor(in_features, num_classes)
# 定义数据加载器、优化器等,开始训练模型
```
2. YOLOv3:YOLOv3是一种基于卷积神经网络的目标检测算法,它比较适合用于对大物体的检测。Python实现代码如下:
```python
import cv2
import numpy as np
import urllib.request
# 加载 YOLOv3 模型
net = cv2.dnn.readNetFromDarknet("yolov3.cfg", "yolov3.weights")
# 定义需要检测的物体类别和阈值等参数
classes = ["person", "car", "truck", ...]
conf_threshold = 0.5
nms_threshold = 0.4
colors = np.random.uniform(0, 255, size=(len(classes), 3))
# 加载测试图片,执行目标检测
img_url = "https://example.com/test.jpg"
urllib.request.urlretrieve(img_url, "test.jpg")
img = cv2.imread("test.jpg")
blob = cv2.dnn.blobFromImage(img, 1/255, (416, 416), swapRB=True, crop=False)
net.setInput(blob)
outs = net.forward(net.getUnconnectedOutLayersNames())
# 预测并绘制边界框
boxes = []
confidences = []
class_ids = []
HT, WT, _ = img.shape
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > conf_threshold:
x, y, w, h = detection[:4] * np.array([WT, HT, WT, HT])
left = int(x - w/2)
top = int(y - h/2)
right = int(x + w/2)
bottom = int(y + h/2)
boxes.append([left, top, right, bottom])
confidences.append(float(confidence))
class_ids.append(int(class_id))
indices = cv2.dnn.NMSBoxes(boxes, confidences, conf_threshold, nms_threshold)
for i in indices:
i = i[0]
box = boxes[i]
left, top, right, bottom = box
label = f"{classes[class_ids[i]]}: {confidences[i]:.2f}"
color = colors[class_ids[i]]
cv2.rectangle(img, (left, top), (right, bottom), color, 2)
cv2.putText(img, label, (left, top-5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, color, 2)
# 显示结果图像
cv2.imshow("result", img)
cv2.waitkey(0)
cv2.destroyAllWindows()
```
以上代码仅仅是快速示例,如果想要更深入地了解这些模型的使用和原理,需要查阅更多资料、学习更多知识。
相关推荐
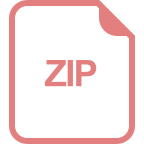
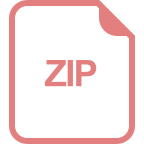
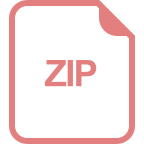
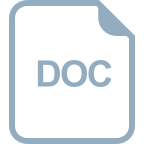
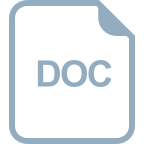
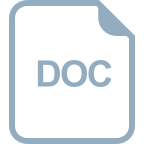
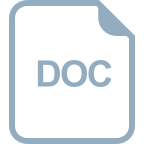
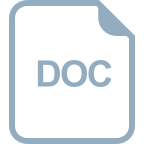
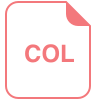








