Abstract Factory Pattern设计模式及C++代码
时间: 2023-02-26 19:48:41 浏览: 57
Abstract Factory Pattern是一种设计模式,它是创建对象的工厂模式的变体,允许对象在运行时被替换。
Abstract Factory模式提供了一种方法,可以创建一组相关或相互依赖的对象,而不需要明确指定其具体类。
下面是一个C语言的代码示例,该代码实现了一个抽象工厂模式,该模式创建一组车辆:
```c
#include <stdio.h>
typedef struct IVehicle IVehicle;
struct IVehicle {
void (*Drive)(IVehicle *);
};
typedef struct Car Car;
struct Car {
IVehicle base;
int wheelCount;
};
void Car_Drive(IVehicle *vehicle) {
Car *car = (Car *)vehicle;
printf("Driving a car with %d wheels\n", car->wheelCount);
}
typedef struct Bike Bike;
struct Bike {
IVehicle base;
int pedalCount;
};
void Bike_Drive(IVehicle *vehicle) {
Bike *bike = (Bike *)vehicle;
printf("Riding a bike with %d pedals\n", bike->pedalCount);
}
typedef struct IVehicleFactory IVehicleFactory;
struct IVehicleFactory {
IVehicle *(*CreateVehicle)(IVehicleFactory *);
};
typedef struct CarFactory CarFactory;
struct CarFactory {
IVehicleFactory base;
};
IVehicle *CarFactory_CreateVehicle(IVehicleFactory *factory) {
Car *car = (Car *)malloc(sizeof(Car));
car->base.Drive = &Car_Drive;
car->wheelCount = 4;
return (IVehicle *)car;
}
typedef struct BikeFactory BikeFactory;
struct BikeFactory {
IVehicleFactory base;
};
IVehicle *BikeFactory_CreateVehicle(IVehicleFactory *factory) {
Bike *bike = (Bike *)malloc(sizeof(Bike));
bike->base.Drive = &Bike_Drive;
bike->pedalCount = 2;
return (IVehicle *)bike;
}
int main(int argc, char *argv[]) {
CarFactory carFactory = { { &CarFactory_CreateVehicle } };
IVehicle *vehicle = carFactory.base.CreateVehicle((IVehicleFactory *)
相关推荐
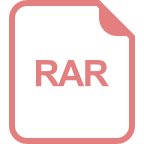
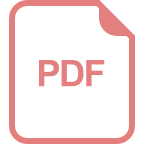
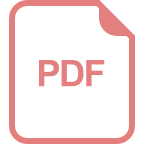












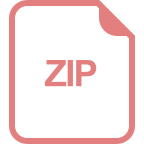
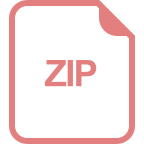
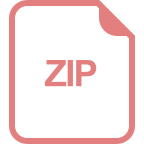