线性代数中的矩阵可以表示为一个row*column的二维数组,当row和column均为1时,退化为一个数,当row为1时,为一个行向量,当column为1时,为一个列向量。 建立一个整数矩阵类matrix,其私有数据成员如下: int row; int column; int **mat; 建立该整数矩阵类matrix构造函数; 建立一个 *(乘号)的运算符重载,以便于对两个输入矩阵进行乘法运算; 建立输出函数void display(),对整数矩阵按行进行列对齐输出,格式化输出语句如下: cout
时间: 2023-07-15 20:13:05 浏览: 223
以下是整数矩阵类matrix的实现代码,包括构造函数、乘法运算符重载和输出函数:
```c++
#include <iostream>
using namespace std;
class matrix {
private:
int row;
int column;
int **mat;
public:
// 构造函数
matrix(int r, int c) {
row = r;
column = c;
mat = new int*[row];
for (int i = 0; i < row; i++) {
mat[i] = new int[column];
}
}
// 乘法运算符重载
matrix operator*(const matrix& other) {
if (column != other.row) {
cout << "Error: Invalid matrix size for multiplication!" << endl;
exit(1);
}
matrix result(row, other.column);
for (int i = 0; i < row; i++) {
for (int j = 0; j < other.column; j++) {
result.mat[i][j] = 0;
for (int k = 0; k < column; k++) {
result.mat[i][j] += mat[i][k] * other.mat[k][j];
}
}
}
return result;
}
// 输出函数
void display() {
for (int i = 0; i < row; i++) {
for (int j = 0; j < column; j++) {
cout << mat[i][j] << " ";
}
cout << endl;
}
}
};
```
使用示例:
```c++
int main() {
matrix A(2, 3);
A.mat[0][0] = 1; A.mat[0][1] = 2; A.mat[0][2] = 3;
A.mat[1][0] = 4; A.mat[1][1] = 5; A.mat[1][2] = 6;
matrix B(3, 2);
B.mat[0][0] = 7; B.mat[0][1] = 8;
B.mat[1][0] = 9; B.mat[1][1] = 10;
B.mat[2][0] = 11; B.mat[2][1] = 12;
matrix C = A * B;
C.display(); // 输出结果为:
// 58 64
// 139 154
return 0;
}
```
阅读全文
相关推荐
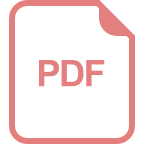
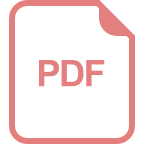
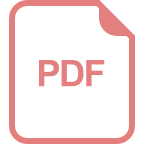















