假设已经定义了Vector.cpp,Vector.h,分别为Vector对象的定义和声明,再定义一个user.cpp,里面定义一个需要用到Vector的函数sqrt_sum(Vector& v),最后,我们在Unit.cpp中创建main函数,并调用sqrt_sum函数
时间: 2024-03-24 20:40:34 浏览: 62
好的,您的问题是如何在Unit.cpp中创建main函数并调用sqrt_sum函数。下面是示例代码:
```c++
// Unit.cpp
#include "Vector.h"
#include "user.h"
int main() {
Vector v(3);
v[0] = 1;
v[1] = 2;
v[2] = 3;
double result = sqrt_sum(v);
cout << "The square root of the sum of the vector is: " << result << endl;
return 0;
}
```
在上面的代码中,我们包含了Vector.h和user.h头文件,并创建了一个大小为3的Vector对象v。然后,我们将其元素赋值为1、2、3。接着,我们调用sqrt_sum函数,并将v作为参数传递给它。最后,我们将返回值输出到控制台上。
请注意,为了能够调用sqrt_sum函数,我们需要在Unit.cpp中包含user.h头文件,因为它包含了函数的声明。另外,为了能够使用Vector类型,我们还需要包含Vector.h头文件。
相关问题
上面的代码不需要#include“user.cpp”吗
不需要在main函数中包含`#include "user.cpp"`,因为在`sqrt_sum`函数定义的文件`Vector.h`中已经包含了所有必要的头文件。只需要在main函数中包含`Vector.h`头文件即可。同时,也不建议在main函数中包含`.cpp`文件,因为`.cpp`文件通常只包含实现代码而不包含声明,这可能会导致重复定义的错误。
写一个.cpp文件,要求如下:可以实现用户的注册登录;将用户信息储存在txt文件中
以下是一个简单的示例代码,实现用户的注册和登录,并将用户信息储存在txt文件中:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
struct User {
string username;
string password;
};
vector<User> users;
void read_users() {
ifstream file("users.txt");
if (!file) {
cerr << "Failed to open file 'users.txt'!" << endl;
return;
}
users.clear();
while (true) {
User user;
if (!(file >> user.username >> user.password)) {
break;
}
users.push_back(user);
}
file.close();
}
void write_users() {
ofstream file("users.txt");
if (!file) {
cerr << "Failed to open file 'users.txt'!" << endl;
return;
}
for (const auto& user : users) {
file << user.username << ' ' << user.password << endl;
}
file.close();
}
bool register_user() {
string username, password;
cout << "Please enter your username: ";
cin >> username;
for (const auto& user : users) {
if (user.username == username) {
cerr << "Username already exists!" << endl;
return false;
}
}
cout << "Please enter your password: ";
cin >> password;
users.push_back({username, password});
write_users();
cout << "User registered successfully!" << endl;
return true;
}
bool login() {
string username, password;
cout << "Please enter your username: ";
cin >> username;
for (const auto& user : users) {
if (user.username == username) {
cout << "Please enter your password: ";
cin >> password;
if (user.password == password) {
cout << "Login successful!" << endl;
return true;
}
else {
cerr << "Incorrect password!" << endl;
return false;
}
}
}
cerr << "Username not found!" << endl;
return false;
}
int main() {
read_users();
while (true) {
cout << "Please choose an action:" << endl;
cout << "1. Register" << endl;
cout << "2. Login" << endl;
cout << "3. Quit" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
register_user();
break;
case 2:
login();
break;
case 3:
return 0;
default:
cerr << "Invalid choice!" << endl;
}
}
}
```
在该代码中,我们定义了一个 `User` 结构体,用于存储用户的用户名和密码。然后,我们使用一个 `vector` 来存储所有的用户信息,并使用 `read_users()` 和 `write_users()` 函数来读取和写入用户信息到文件中。
在 `register_user()` 函数中,我们首先要求用户输入他们的用户名和密码。然后,我们检查是否有其他用户使用了相同的用户名。如果是,则返回错误。否则,我们将新用户的信息添加到 `users` 向量中,并将所有用户信息写入文件中。
在 `login()` 函数中,我们要求用户输入他们的用户名和密码。然后,我们检查是否有已注册的用户使用了相同的用户名。如果是,则检查输入的密码是否与该用户匹配。如果密码正确,则返回成功。否则,返回错误。
在 `main()` 函数中,我们使用一个循环来不断地要求用户选择一个操作。如果用户选择注册或登录,则调用相应的函数。如果用户选择退出,则退出程序。
注意:上述示例代码仅供参考,实际应用中可能需要更复杂的安全措施以确保用户信息的安全性。
阅读全文
相关推荐
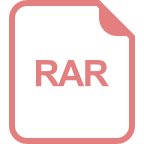
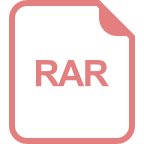
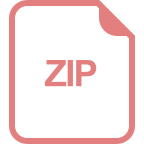













