应用顺序栈,编写程序实现回文字符串判定。
时间: 2024-03-16 10:47:01 浏览: 61
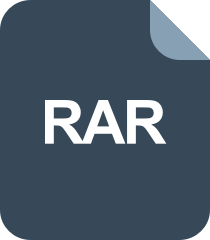
4.4.1.rar_数据结构_Visual_C++_
好的,我可以为你编写这个程序。以下是使用顺序栈判断回文字符串的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MaxSize 100
typedef struct {
char data[MaxSize];
int top;
} SqStack;
// 初始化栈
void InitStack(SqStack *S) {
S->top = -1;
}
// 判断栈是否为空
int StackEmpty(SqStack S) {
if (S.top == -1) {
return 1;
} else {
return 0;
}
}
// 入栈
int Push(SqStack *S, char x) {
if (S->top == MaxSize - 1) {
return 0;
}
S->top++;
S->data[S->top] = x;
return 1;
}
// 出栈
int Pop(SqStack *S, char *x) {
if (S->top == -1) {
return 0;
}
*x = S->data[S->top];
S->top--;
return 1;
}
// 判断字符串是否为回文
int IsPalindrome(char *str) {
SqStack S;
InitStack(&S);
int len = strlen(str);
int i;
for (i = 0; i < len / 2; i++) {
Push(&S, str[i]);
}
if (len % 2 != 0) {
i++;
}
char x;
while (i < len) {
Pop(&S, &x);
if (x != str[i]) {
return 0;
}
i++;
}
return 1;
}
int main() {
char str[MaxSize];
printf("请输入一个字符串:");
scanf("%s", str);
if (IsPalindrome(str)) {
printf("%s 是回文字符串\n", str);
} else {
printf("%s 不是回文字符串\n", str);
}
return 0;
}
```
这个程序使用顺序栈来判断字符串是否为回文字符串。首先将字符串的前一半字符入栈,然后依次弹出栈顶字符与后一半字符比较,如果相同则继续比较,否则说明字符串不是回文字符串。程序中定义了一个 SqStack 结构体,包含一个字符数组和一个整型变量 top,用于表示栈顶元素的下标。程序中还包含了一些栈的基本操作函数,如 InitStack、StackEmpty、Push 和 Pop。最后在主函数中调用了 IsPalindrome 函数来判断输入的字符串是否为回文字符串。
阅读全文
相关推荐
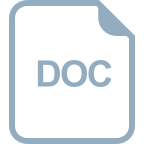
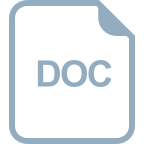

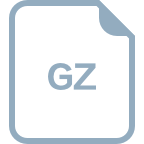
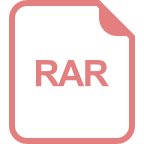






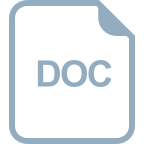
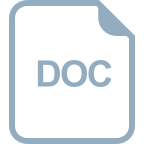