public void Up(){ boolean flag=false; for(int y=0;y<4;y++){ for(int x=0;x<4;x++){ for(int x1=x+1;x1<4;x1++){ if(card[x1][y].getText()!=""){ int num = Integer.parseInt(card[x1][y].getText().toString()); if(card[x][y].getText()==""){ card[x][y].setText(num+""); card[x1][y].setText(""); x--; flag=true; break; }else if(equals(card[x][y],card[x1][y])){ num*=2; card[x][y].setText(num+""); card[x1][y].setText(""); int score = Integer.parseInt(tvScore.getText().toString()); score+=num; tvScore.setText(score+""); flag=true; break; }else{ break; } } } } } if(flag) { RandNum(); StopGame(); } }
时间: 2023-06-19 21:07:56 浏览: 88
这段代码是实现 2048 游戏中数字方块上滑动的方法,具体的实现细节如下:
1. 首先设置一个标志变量 flag,用来记录本轮操作是否有方块发生移动。
2. 外层循环遍历每一列,内层循环遍历每一行,然后再次内层循环遍历每一行中当前行后面的所有行。
3. 如果当前行后面的一行中有数字方块,则执行以下操作:
a. 将当前行后面的方块的数字 num 转化为 int 类型。
b. 如果当前行中的方块为空,则将数字方块移动到当前行,将当前行后面的方块清空,并将标志变量 flag 设置为 true。
c. 如果当前行中的方块与后面的方块数字相同,则将两个数字相加,将结果放在当前行中,将后面的方块清空,并将标志变量 flag 设置为 true。
d. 如果当前行中的方块与后面的方块数字不同,则不做任何处理,直接跳出当前循环。
4. 如果本轮操作有方块发生移动,则生成一个新的数字方块,检查是否游戏结束,并刷新分数。
相关问题
public void Down(){ boolean flag=false; for(int y=0;y<4;y++){ for(int x=3;x>=0;x--){ for(int x1=x-1;x1>=0;x1--){ if(card[x1][y].getText()!=""){ int num = Integer.parseInt(card[x1][y].getText().toString()); if(card[x][y].getText()==""){ card[x][y].setText(num+""); card[x1][y].setText(""); x++; flag=true; break; }else if(equals(card[x][y],card[x1][y])){ num*=2; card[x][y].setText(num+""); card[x1][y].setText(""); int score = Integer.parseInt(tvScore.getText().toString()); score+=num; tvScore.setText(score+""); flag=true; break; }else{ break; } } } } } if(flag) { RandNum(); StopGame(); } }
这是一个2048小游戏的下移函数。这个函数的作用是将所有的数字卡片向下移动,当两个相邻的卡片数字相同时,它们会合并成一个新的卡片,数字为原来两个卡片数字的和。同时,每次移动和合并都会增加分数。
具体实现方式是,从第一列开始,从下往上遍历每个格子,找到它上面第一个不为空的格子(即其上面有数字的格子),如果这个格子和它自己数字相同,则将两个格子合并,并更新分数;否则,将这个格子移动到上面第一个空格子的位置。每次操作后,如果有格子发生了移动或合并,就随机生成一个新的数字卡片,并检查游戏是否结束。
import java.util.Scanner; public class PTA01 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int n = Integer.parseInt(sc.nextLine()); String input = sc.nextLine(); String[] strarr = input.split(" "); int[] arr = new int[n]; for (int i = 0; i < n; i++) { arr[i] = Integer.parseInt(strarr[i]); } SeceltSort(arr); } public static void SeceltSort(int[] arr) { for (int i = 0; i < arr.length; i++) { int temp = arr[i]; int index = i; boolean flag = false; for (int j = i; j < arr.length; j++) { if (temp > arr[j]) { temp = arr[j]; index = j; flag = true; } } if (flag == false) continue; temp = arr[i]; arr[i] = arr[index]; arr[index] = temp; if(i!=0) System.out.println(); for (int a = 0; a < arr.length; a++) { System.out.print(arr[a]); if (a!=arr.length-1) System.out.print(" "); } } } }
这段代码实现的是选择排序,输入一个整数 n 和 n 个整数,然后对这 n 个整数进行选择排序并输出每次排序后的结果。
具体实现过程:
首先通过 Scanner 对象读取输入,第一行是整数 n,第二行是 n 个整数,用 split 方法将第二行的字符串按空格分割成整数数组。
然后调用 SeceltSort 方法进行选择排序,遍历数组,每次找到未排序部分的最小值,将其与未排序部分的第一个元素交换位置。在交换位置后,输出当前排序结果。
最后的输出结果类似于:
1 2 3 4 5
1 2 3 4 5
1 2 3 4 5
1 2 3 4 5
1 2 3 4 5
每行输出一个排序结果。
阅读全文
相关推荐
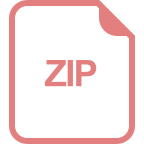
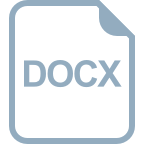
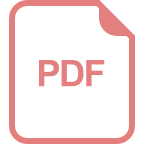













