设计一个二维点类,从二维点类派生出三维点类设计访问相关属性的方法以及计算点到坐标原点距离的方法(c++)
时间: 2024-03-08 15:46:19 浏览: 63
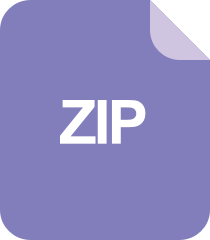
C++实现三维空间中点到点、点到直线、点到平面的距离计算

好的,以下是设计的代码示例(使用C++语言):
```c++
#include<iostream>
#include<cmath>
using namespace std;
class Point2D {
protected:
double x;
double y;
public:
Point2D(double x, double y) {
this->x = x;
this->y = y;
}
double get_x() {
return x;
}
double get_y() {
return y;
}
double distance_to_origin() {
return sqrt(x * x + y * y);
}
};
class Point3D : public Point2D {
private:
double z;
public:
Point3D(double x, double y, double z) : Point2D(x, y) {
this->z = z;
}
double get_z() {
return z;
}
double distance_to_origin() {
return sqrt(x * x + y * y + z * z);
}
};
int main() {
Point2D p2d(3.0, 4.0);
Point3D p3d(3.0, 4.0, 5.0);
cout << "2D point: (" << p2d.get_x() << ", " << p2d.get_y() << ")" << endl;
cout << "Distance to origin: " << p2d.distance_to_origin() << endl;
cout << "3D point: (" << p3d.get_x() << ", " << p3d.get_y() << ", " << p3d.get_z() << ")" << endl;
cout << "Distance to origin: " << p3d.distance_to_origin() << endl;
return 0;
}
```
以上代码定义了一个二维点类`Point2D`和一个三维点类`Point3D`,其中三维点类`Point3D`继承了二维点类`Point2D`的属性和方法。`Point2D`类有`x`和`y`两个属性,可以通过`get_x()`和`get_y()`方法获取这两个属性的值。`distance_to_origin()`方法可以计算该点到坐标原点的距离。
`Point3D`类除了继承了`Point2D`类的属性和方法外,还有一个`z`属性,可以通过`get_z()`方法获取该属性的值。覆盖了父类的`distance_to_origin()`方法,可以计算该点到坐标原点的距离。
这样设计的好处是,我们可以根据需要方便地扩展出更多的点类,而且不同类的实例可以调用相同的方法名,实现了代码的重用。
阅读全文
相关推荐







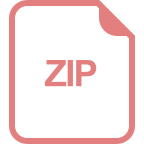







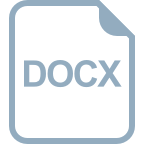