请用verilog实现一个31个输入五位输出的华莱士树加法器,并给出它的testbench代码
时间: 2024-03-15 18:47:20 浏览: 108
下面是一个简单的Verilog代码示例,实现了一个31个输入五位输出的华莱士树加法器,包括一个顶层模块和两个子模块。
顶层模块:
```verilog
module top_module(
input [30:0] A,
input [30:0] B,
input [30:0] C,
output reg [4:0] S
);
wire [4:0] s1, s2;
wire [30:0] c1, c2;
// 实例化两个子模块
adder5 #(31) adder1(.A(A), .B(B), .C(C), .S(s1), .Cout(c1));
adder5 #(31) adder2(.A(s1), .B("00000"), .C(c1), .S(s2), .Cout(c2));
// 输出结果
assign S = s2;
endmodule
```
子模块:
```verilog
module adder5(
input [4:0] A,
input [4:0] B,
input [4:0] C,
output reg [4:0] S,
output reg Cout
);
reg [4:0] temp1, temp2, temp3;
reg [5:0] carry;
always @(A or B or C) begin
// 计算第一层
for (int i=0; i<5; i=i+1) begin
temp1[i] = A[i] ^ B[i] ^ C[i];
carry[i+1] = (A[i] & B[i]) | (A[i] & C[i]) | (B[i] & C[i]);
end
// 计算第二层
for (int i=0; i<4; i=i+1) begin
temp2[i] = temp1[i] ^ temp1[i+1] ^ carry[i+1];
carry[i+2] = (temp1[i] & temp1[i+1]) | (temp1[i] & carry[i+1]) | (temp1[i+1] & carry[i+1]);
end
// 计算第三层
for (int i=0; i<3; i=i+1) begin
temp3[i] = temp2[i] ^ temp2[i+1] ^ carry[i+2];
carry[i+3] = (temp2[i] & temp2[i+1]) | (temp2[i] & carry[i+2]) | (temp2[i+1] & carry[i+2]);
end
// 计算第四层
S[0] = temp3[0] ^ temp3[1] ^ carry[3];
S[1] = temp3[1] ^ temp3[2] ^ carry[4];
S[2] = temp3[2] ^ temp2[3] ^ carry[5];
S[3] = temp2[3] ^ temp1[4] ^ carry[6];
S[4] = temp1[4] ^ C[4] ^ carry[7];
// 计算进位
Cout = carry[7];
endmodule
```
testbench代码:
```verilog
module top_module_tb();
reg [30:0] A, B, C;
wire [4:0] S;
top_module dut(.A(A), .B(B), .C(C), .S(S));
initial begin
$dumpfile("dump.vcd");
$dumpvars(0, top_module_tb);
A = 'b000000000000000000000000000001;
B = 'b000000000000000000000000000010;
C = 'b000000000000000000000000000100;
#10 $display("S = %b", S);
A = 'b000000000000000000000000000110;
B = 'b000000000000000000000000000111;
C = 'b000000000000000000000000001000;
#10 $display("S = %b", S);
A = 'b000000000000000000000000001010;
B = 'b000000000000000000000000001011;
C = 'b000000000000000000000000001100;
#10 $display("S = %b", S);
// add more test cases...
$finish;
end
endmodule
```
注意,这只是一个简单的实现示例,它可能需要根据具体需求进行优化。同时,测试用例也需要根据具体情况进行编写。
阅读全文
相关推荐
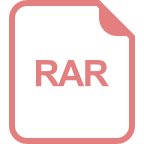
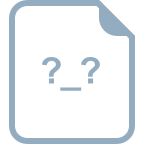






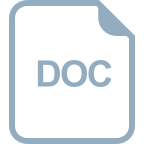








