创建一个简单的javaweb项目能实现分页功能,在jsp页面中有一个表格显示id,name,age这三种信息
时间: 2023-12-14 08:36:27 浏览: 104
好的,下面是一个简单的javaweb项目,实现了分页功能,并在jsp页面中显示了id、name和age三种信息。
首先,我们需要创建一个Student类,用于表示学生信息:
```
public class Student {
private int id;
private String name;
private int age;
// 构造函数和getter/setter方法省略
}
```
然后,我们需要创建一个Dao类,用于从数据库中获取学生信息。在这个例子中,我们使用MySQL数据库,并且假设有一个students表存储学生信息。
```
public class StudentDao {
private static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
private static final String DB_URL = "jdbc:mysql://localhost:3306/test";
private static final String USER = "root";
private static final String PASSWORD = "123456";
public List<Student> getStudents(int start, int count) {
List<Student> students = new ArrayList<Student>();
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASSWORD);
stmt = conn.createStatement();
String sql = "SELECT id, name, age FROM students LIMIT " + start + ", " + count;
rs = stmt.executeQuery(sql);
while (rs.next()) {
Student student = new Student();
student.setId(rs.getInt("id"));
student.setName(rs.getString("name"));
student.setAge(rs.getInt("age"));
students.add(student);
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) rs.close();
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return students;
}
}
```
接下来,我们需要创建一个Servlet类,用于接收分页请求,并调用Dao类获取学生信息。在这个例子中,我们使用GET请求,并且假设每页显示10个学生信息。
```
@WebServlet("/student")
public class StudentServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private static final int PAGE_SIZE = 10;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String pageStr = request.getParameter("page");
int page = pageStr == null ? 1 : Integer.parseInt(pageStr);
int start = (page - 1) * PAGE_SIZE;
int count = PAGE_SIZE;
StudentDao dao = new StudentDao();
List<Student> students = dao.getStudents(start, count);
request.setAttribute("students", students);
request.setAttribute("page", page);
RequestDispatcher rd = request.getRequestDispatcher("/student.jsp");
rd.forward(request, response);
}
}
```
最后,我们需要创建一个jsp页面,用于显示学生信息并提供分页导航。
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Student List</title>
</head>
<body>
<h1>Student List</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<c:forEach var="student" items="${students}">
<tr>
<td>${student.id}</td>
<td>${student.name}</td>
<td>${student.age}</td>
</tr>
</c:forEach>
</tbody>
</table>
<div>
<c:if test="${page > 1}">
<a href="${page - 1}">Prev</a>
</c:if>
<c:if test="${not empty students}">
<a href="${page + 1}">Next</a>
</c:if>
</div>
</body>
</html>
```
在这个例子中,我们使用了JSTL标签库来遍历学生信息和生成分页导航。注意,我们使用`${page - 1}`和`${page + 1}`来生成上一页和下一页的链接,并且需要判断当前页是否为第一页或最后一页。
阅读全文
相关推荐
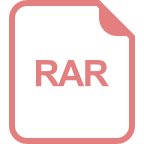
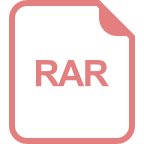
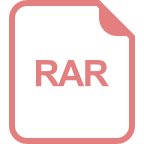















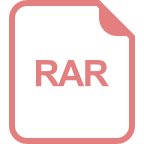