设计一个算法,实现将顺序表就地逆置,即利用原顺序表的存储单元将数据元素序列(a0,a1,…,an-1)逆置为(an-1,…, a1,a0)。
时间: 2024-05-06 09:20:36 浏览: 89
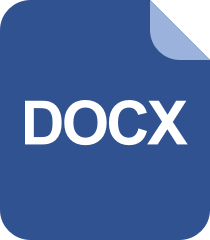
顺序表就地逆置
算法步骤:
1.定义两个指针,分别指向顺序表的第一个元素和最后一个元素。
2.交换两个指针所指向的元素,并将两个指针向中间移动,直到两个指针相遇或交叉。
3.顺序表就地逆置完成。
算法实现:
```python
def reverse(lst):
left, right = 0, len(lst)-1 # 定义左右指针
while left < right:
lst[left], lst[right] = lst[right], lst[left] # 交换元素
left += 1
right -= 1 # 移动指针
```
测试:
```python
lst = [1, 2, 3, 4, 5]
reverse(lst)
print(lst) # 输出 [5, 4, 3, 2, 1]
```
阅读全文
相关推荐
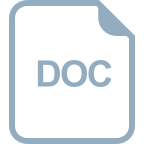
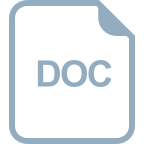















