不要使用库用C语言写Modbus协议主站
时间: 2023-07-20 10:30:48 浏览: 99
好的,我来简单介绍一下如何使用C语言编写Modbus协议主站。
Modbus是一种串行通信协议,用于在工业控制系统中传输数据。在Modbus协议中,主站负责发送请求,从站则负责响应请求。以下是一个简单的C语言程序,实现了Modbus协议主站的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <errno.h>
#include <fcntl.h>
#include <termios.h>
#define SLAVE_ADDRESS 0x01
#define FUNCTION_CODE 0x03
#define START_ADDRESS 0x0000
#define DATA_LENGTH 0x0002
int main(int argc, char *argv[])
{
int fd;
struct termios options;
unsigned char request[8] = { SLAVE_ADDRESS, FUNCTION_CODE, START_ADDRESS >> 8, START_ADDRESS & 0xFF, DATA_LENGTH >> 8, DATA_LENGTH & 0xFF, 0, 0 };
unsigned char response[256];
// 打开串口设备
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd < 0) {
perror("open");
exit(1);
}
// 配置串口参数
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cc[VMIN] = 1;
options.c_cc[VTIME] = 0;
tcsetattr(fd, TCSANOW, &options);
// 发送请求
write(fd, request, sizeof(request));
// 等待响应
usleep(10000);
int count = read(fd, response, sizeof(response));
if (count < 0) {
perror("read");
exit(1);
}
// 处理响应
printf("Response: ");
for (int i = 0; i < count; i++) {
printf("%02X ", response[i]);
}
printf("\n");
// 关闭串口设备
close(fd);
return 0;
}
```
在这个程序中,我们首先定义了从站地址、功能码、起始地址和数据长度等参数。然后打开串口设备,配置串口参数。
接着,我们组装了一个请求报文,并通过串口发送出去。然后等待从站响应,读取响应报文。最后,我们可以处理响应报文,打印出每一个字节的值。
需要注意的是,这个程序只是一个简单的例子,实际的Modbus协议主站可能需要处理更加复杂的请求和响应报文。同时,我们也需要根据具体的硬件和系统配置来配置串口参数,保证程序可以正常工作。
希望这个简单的例子可以帮助你了解如何使用C语言编写Modbus协议主站。
阅读全文
相关推荐
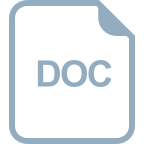
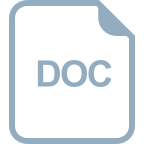
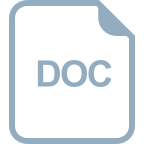
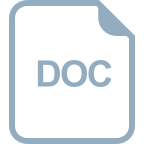

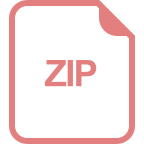
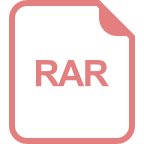
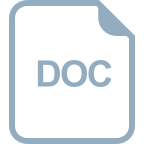
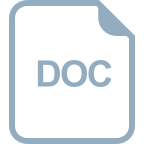
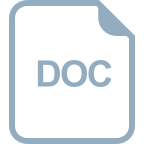
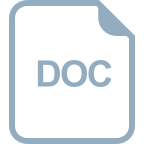
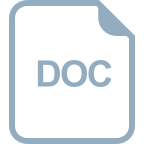
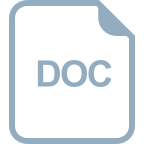
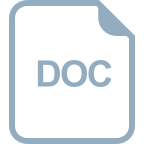
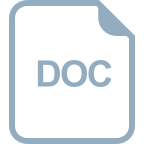




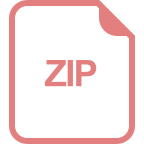