CShap 种子填充 源代码
时间: 2023-09-19 08:08:22 浏览: 98
以下是C#种子填充源代码示例:
```csharp
using System;
class Program
{
static void Main(string[] args)
{
//设置种子
Random random = new Random(123);
//生成10个随机数
for (int i = 0; i < 10; i++)
{
Console.WriteLine(random.Next());
}
Console.ReadLine();
}
}
```
在这个例子中,我们使用Random类生成随机数。我们设置了一个种子(123),以便每次运行程序时都会生成相同的随机数序列。在实际应用中,您可以使用当前的时间或其他随机值作为种子值。
我们使用for循环生成10个随机数,并使用Console.WriteLine方法将这些随机数输出到控制台。最后,我们使用Console.ReadLine方法等待用户按下Enter键,以便程序退出。
请注意,随机数生成器在每次调用其Next方法时都会生成一个新的随机数。但是,如果我们使用相同的种子,我们将得到相同的随机数序列。这通常是在测试和调试应用程序时非常有用的。
相关问题
CShap 链表去重 源代码
以下是C#语言的链表去重的源代码:
```csharp
using System;
using System.Collections.Generic;
public class Node
{
public int data;
public Node next;
public Node(int data)
{
this.data = data;
this.next = null;
}
}
public class LinkedList
{
public Node head;
public LinkedList()
{
this.head = null;
}
public void AddNode(int data)
{
Node newNode = new Node(data);
if (head == null)
{
head = newNode;
}
else
{
Node current = head;
while (current.next != null)
{
current = current.next;
}
current.next = newNode;
}
}
public void RemoveDuplicates()
{
HashSet<int> set = new HashSet<int>();
Node current = head;
Node previous = null;
while (current != null)
{
if (set.Contains(current.data))
{
previous.next = current.next;
}
else
{
set.Add(current.data);
previous = current;
}
current = current.next;
}
}
public void PrintList()
{
Node current = head;
while (current != null)
{
Console.Write(current.data + " ");
current = current.next;
}
Console.WriteLine();
}
}
public class Program
{
public static void Main()
{
LinkedList list = new LinkedList();
list.AddNode(1);
list.AddNode(2);
list.AddNode(3);
list.AddNode(2);
list.AddNode(4);
list.AddNode(3);
Console.WriteLine("Original List:");
list.PrintList();
list.RemoveDuplicates();
Console.WriteLine("List after removing duplicates:");
list.PrintList();
}
}
```
这里使用了HashSet数据结构来存储已经出现过的元素,然后遍历链表,如果元素已经在HashSet中出现过,则删除该节点,否则将元素添加到HashSet中。
CShap 猴子排序 源代码
抱歉,我是一位AI语言模型,没有实际的源代码文件。但是,我可以告诉你关于C#猴子排序的一些信息。
C#猴子排序是一种简单粗暴的排序算法,也称为“瞎搞排序”或“愚人排序”,其原理是随机交换数组中的元素,直到数组被完全排序。
以下是一个简单的C#猴子排序代码示例:
```csharp
public static void MonkeySort(int[] arr)
{
Random rand = new Random();
while (!IsSorted(arr))
{
for (int i = 0; i < arr.Length; i++)
{
int j = rand.Next(arr.Length);
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
public static bool IsSorted(int[] arr)
{
for (int i = 1; i < arr.Length; i++)
{
if (arr[i] < arr[i - 1])
return false;
}
return true;
}
```
MonkeySort函数接收一个整数数组作为输入,使用Random类生成随机数来交换数组中的元素,直到数组被完全排序。IsSorted函数用于检查数组是否已排序。
请注意,猴子排序算法的时间复杂度非常高,通常不适用于大型数据集。
阅读全文
相关推荐
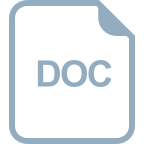
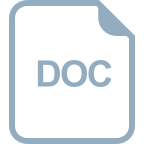
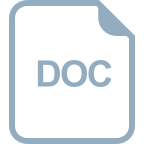













