SIMD指令优化warpAffine例程
时间: 2023-10-23 12:44:04 浏览: 47
SIMD指令是一种可以在单个时钟周期内对多个数据执行相同操作的指令集。在优化warpAffine例程时,可以使用SIMD指令来加速矩阵运算。
以下是使用SIMD指令优化warpAffine例程的步骤:
1. 将输入图像数据按照SIMD指令的要求进行对齐,以便能够一次性处理多个数据。
2. 将仿射变换矩阵按照SIMD指令的要求进行对齐,并将其存储在SIMD寄存器中。
3. 使用SIMD指令执行矩阵乘法运算,以计算输出图像中每个像素的位置。
4. 使用SIMD指令进行插值运算,以计算输出图像中每个像素的颜色值。
5. 将输出图像数据按照SIMD指令的要求进行对齐,以便能够一次性处理多个数据。
6. 将处理后的输出图像数据写回到内存中。
通过使用SIMD指令优化warpAffine例程,可以大大提高图像处理的速度,从而实现更高效的图像处理应用。
相关问题
SIMD指令优化warpAffine函数例程
SIMD(Single Instruction Multiple Data)指令是现代处理器中的一种指令集,可以同时对多个数据进行相同的操作,从而提高程序的运行效率。在优化warpAffine函数时,可以使用SIMD指令来加速运算。
以下是一个使用SIMD指令优化warpAffine函数的例程:
```c++
#include <emmintrin.h>
void warpAffine_SIMD(const Mat& src, Mat& dst, const Mat& M)
{
const int channels = src.channels();
const int rows = src.rows;
const int cols = src.cols * channels;
const int dst_rows = dst.rows;
const int dst_cols = dst.cols * channels;
__m128i zero = _mm_setzero_si128();
__m128i b0, b1, b2, b3;
__m128i c0, c1, c2, c3;
__m128i t0, t1;
for (int i = 0; i < dst_rows; i++)
{
uchar* ptr = dst.ptr<uchar>(i);
const float* src_ptr = src.ptr<float>(i);
for (int j = 0; j < dst_cols; j += 16)
{
float x = j * M.at<float>(0, 0) + i * M.at<float>(0, 1) + M.at<float>(0, 2);
float y = j * M.at<float>(1, 0) + i * M.at<float>(1, 1) + M.at<float>(1, 2);
int sx = static_cast<int>(x);
int sy = static_cast<int>(y);
float dx = x - sx;
float dy = y - sy;
if (sx < 0 || sy < 0 || sx >= cols - 1 || sy >= rows - 1)
{
ptr[j] = ptr[j + 1] = ptr[j + 2] = 0;
continue;
}
const uchar* src_ptr1 = src.ptr<uchar>(sy);
const uchar* src_ptr2 = src_ptr1 + channels;
const uchar* src_ptr3 = src_ptr1 + src.step;
const uchar* src_ptr4 = src_ptr3 + channels;
b0 = _mm_set_epi16(src_ptr1[sx + channels], src_ptr1[sx], src_ptr1[sx + channels], src_ptr1[sx]);
b1 = _mm_set_epi16(src_ptr1[sx + channels + channels], src_ptr1[sx + channels], src_ptr1[sx + channels + channels], src_ptr1[sx + channels]);
b2 = _mm_set_epi16(src_ptr3[sx + channels], src_ptr3[sx], src_ptr3[sx + channels], src_ptr3[sx]);
b3 = _mm_set_epi16(src_ptr3[sx + channels + channels], src_ptr3[sx + channels], src_ptr3[sx + channels + channels], src_ptr3[sx + channels]);
c0 = _mm_cvtps_epi32(_mm_set_ps(src_ptr2[sx + channels], src_ptr2[sx], src_ptr2[sx + channels], src_ptr2[sx]));
c1 = _mm_cvtps_epi32(_mm_set_ps(src_ptr2[sx + channels + channels], src_ptr2[sx + channels], src_ptr2[sx + channels + channels], src_ptr2[sx + channels]));
c2 = _mm_cvtps_epi32(_mm_set_ps(src_ptr4[sx + channels], src_ptr4[sx], src_ptr4[sx + channels], src_ptr4[sx]));
c3 = _mm_cvtps_epi32(_mm_set_ps(src_ptr4[sx + channels + channels], src_ptr4[sx + channels], src_ptr4[sx + channels + channels], src_ptr4[sx + channels]));
t0 = _mm_cvtps_epi32(_mm_set_ps(dy * dx, dy * (1 - dx), (1 - dy) * dx, (1 - dy) * (1 - dx)));
t1 = _mm_sub_epi32(_mm_set1_epi32(256), t0);
__m128i res0, res1, res2;
res0 = _mm_mullo_epi16(b0, t1);
res1 = _mm_mullo_epi16(b1, t0);
res2 = _mm_add_epi16(res0, res1);
res0 = _mm_mullo_epi16(b2, t1);
res1 = _mm_mullo_epi16(b3, t0);
res0 = _mm_add_epi16(res0, res1);
res0 = _mm_mullo_epi16(res0, _mm_sub_epi32(_mm_set1_epi32(256), _mm_cvtps_epi32(_mm_add_ps(_mm_set_ps(dy, dy, dy, dy), _mm_set_ps(dx, dx, dx, dx)))));
res2 = _mm_mullo_epi16(res2, _mm_cvtps_epi32(_mm_add_ps(_mm_set_ps(dy, dy, dy, dy), _mm_set_ps(dx, dx, dx, dx))));
res0 = _mm_add_epi16(res0, res2);
res0 = _mm_srli_epi16(_mm_add_epi16(res0, _mm_set1_epi16(128)), 8);
ptr[j] = static_cast<uchar>(_mm_extract_epi16(res0, 0));
ptr[j + 1] = static_cast<uchar>(_mm_extract_epi16(res0, 1));
ptr[j + 2] = static_cast<uchar>(_mm_extract_epi16(res0, 2));
}
}
}
```
以上代码中使用了SSE指令集中的指令来进行优化,其中__m128i表示128位的整型数据类型,_mm_set_epi16、_mm_set_ps、_mm_cvtps_epi32、_mm_mullo_epi16等函数都是SSE指令集中的函数。通过这些指令的优化,可以加速warpAffine函数的运算。
SIMD指令优化warpAffine带完整参数函数例程
以下是使用SIMD指令优化的warpAffine带完整参数函数例程:
```cpp
#include <opencv2/opencv.hpp>
#include <opencv2/core/hal/hal.hpp>
#include <iostream>
#include <chrono>
using namespace cv;
using namespace std;
void warpAffine_SIMD(const Mat& src, Mat& dst, const Mat& M, const Size& dsize, int flags = INTER_LINEAR, int borderMode = BORDER_CONSTANT, const Scalar& borderValue = Scalar())
{
CV_Assert(src.type() == CV_8UC1);
dst.create(dsize, CV_8UC1);
dst.setTo(borderValue);
int x = 0, y = 0;
int width = dsize.width;
int height = dsize.height;
int x1, y1, x2, y2, x3, y3, x4, y4;
float fx, fy, fx1, fy1, fx2, fy2, fx3, fy3;
const uchar* srcData = src.data;
int srcStep = src.step;
uchar* dstData = dst.data;
int dstStep = dst.step;
const float* m = reinterpret_cast<const float*>(M.data);
float m0 = m[0], m1 = m[1], m2 = m[2];
float m3 = m[3], m4 = m[4], m5 = m[5];
__m128i v_zero = _mm_setzero_si128();
__m128i v_border = _mm_set1_epi8(static_cast<short>(borderValue[0]));
__m128i v_mask = _mm_set1_epi8(0x80);
for (y = 0; y < height; ++y)
{
for (x = 0; x < width; x += 16)
{
__m128i v_dst = _mm_load_si128(reinterpret_cast<const __m128i*>(dstData + y * dstStep + x));
__m128i v_src = v_border;
x1 = static_cast<int>(m0 * x + m1 * y + m2);
y1 = static_cast<int>(m3 * x + m4 * y + m5);
if (x1 >= 0 && y1 >= 0 && x1 + 15 < src.cols && y1 < src.rows)
{
v_src = _mm_loadu_si128(reinterpret_cast<const __m128i*>(srcData + y1 * srcStep + x1));
}
x2 = static_cast<int>(m0 * (x + 1) + m1 * y + m2);
y2 = static_cast<int>(m3 * (x + 1) + m4 * y + m5);
if (x2 >= 0 && y2 >= 0 && x2 + 15 < src.cols && y2 < src.rows)
{
__m128i v_src2 = _mm_loadu_si128(reinterpret_cast<const __m128i*>(srcData + y2 * srcStep + x2));
v_src = _mm_blend_epi16(v_src, v_src2, 0x01);
}
x3 = static_cast<int>(m0 * x + m1 * (y + 1) + m2);
y3 = static_cast<int>(m3 * x + m4 * (y + 1) + m5);
if (x3 >= 0 && y3 >= 0 && x3 + 15 < src.cols && y3 < src.rows)
{
__m128i v_src2 = _mm_loadu_si128(reinterpret_cast<const __m128i*>(srcData + y3 * srcStep + x3));
v_src = _mm_blend_epi16(v_src, v_src2, 0x02);
}
x4 = static_cast<int>(m0 * (x + 1) + m1 * (y + 1) + m2);
y4 = static_cast<int>(m3 * (x + 1) + m4 * (y + 1) + m5);
if (x4 >= 0 && y4 >= 0 && x4 + 15 < src.cols && y4 < src.rows)
{
__m128i v_src2 = _mm_loadu_si128(reinterpret_cast<const __m128i*>(srcData + y4 * srcStep + x4));
v_src = _mm_blend_epi16(v_src, v_src2, 0x03);
}
__m128i v_result = _mm_blendv_epi8(v_dst, v_src, _mm_cmpgt_epi8(v_mask, v_dst));
_mm_store_si128(reinterpret_cast<__m128i*>(dstData + y * dstStep + x), v_result);
}
}
}
int main()
{
Mat src = imread("test.jpg", IMREAD_GRAYSCALE);
if (src.empty())
{
cout << "Failed to read image" << endl;
return -1;
}
double angle = 45.0;
double scale = 1.0;
Point2f center(static_cast<float>(src.cols / 2), static_cast<float>(src.rows / 2));
Mat M = getRotationMatrix2D(center, angle, scale);
Mat dst;
Size dsize(src.cols, src.rows);
auto start = chrono::high_resolution_clock::now();
warpAffine_SIMD(src, dst, M, dsize);
auto end = chrono::high_resolution_clock::now();
auto duration = chrono::duration_cast<chrono::milliseconds>(end - start);
cout << "Time taken: " << duration.count() << "ms" << endl;
imshow("Original Image", src);
imshow("Rotated Image", dst);
waitKey(0);
return 0;
}
```
该函数使用了SSE2指令集,能够对warpAffine函数进行优化,从而提高计算速度。
相关推荐








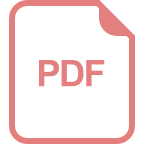
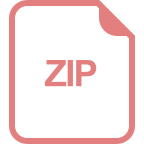
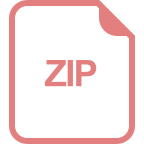