如何使用java httpclient 异步调用https接口
时间: 2023-12-19 11:06:28 浏览: 48
您可以使用Java HttpAsyncClient库来实现异步调用HTTPS接口。HttpAsyncClient库是Apache HttpComponents项目的一部分,它提供了异步的HTTP请求和响应处理。您可以使用SSLContext来设置HTTPS连接。具体的代码实现可以参考HttpAsyncClient官方文档或者相关的教程。
相关问题
如何使用java httpclient 异步调用接口
使用Java HttpClient异步调用接口,可以通过创建异步请求配置对象,设置回调函数并执行异步请求来实现。具体步骤如下:
1.创建异步请求配置对象:
```java
RequestConfig requestConfig = RequestConfig.custom().setSocketTimeout(5000).setConnectTimeout(5000).build();
```
2.创建异步请求执行器:
```java
CloseableHttpAsyncClient httpclient = HttpAsyncClients.createDefault();
httpclient.start();
```
3.创建异步请求对象:
```java
HttpUriRequest request = RequestBuilder.get().setUri("http://www.example.com/").setConfig(requestConfig).build();
```
4.设置回调函数:
```java
Future<HttpResponse> future = httpclient.execute(request, new FutureCallback<HttpResponse>() {
@Override
public void completed(final HttpResponse response) {
System.out.println(request.getRequestLine() + "->" + response.getStatusLine());
}
@Override
public void failed(final Exception ex) {
System.out.println(request.getRequestLine() + "->" + ex);
}
@Override
public void cancelled() {
System.out.println(request.getRequestLine() + " cancelled");
}
});
```
5.执行异步请求:
```java
httpclient.execute(request, future);
```
这样就可以使用Java HttpClient异步调用接口了。
java使用httpclient异步调用webservice的代码
以下是使用Apache HttpClient异步调用WebService的Java代码示例:
```java
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
import javax.xml.namespace.QName;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.soap.MessageFactory;
import javax.xml.soap.MimeHeaders;
import javax.xml.soap.SOAPBody;
import javax.xml.soap.SOAPException;
import javax.xml.soap.SOAPHeader;
import javax.xml.soap.SOAPMessage;
import javax.xml.transform.TransformerException;
import javax.xml.transform.TransformerFactoryConfigurationError;
import org.apache.commons.io.IOUtils;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.concurrent.FutureCallback;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.nio.client.CloseableHttpAsyncClient;
import org.apache.http.impl.nio.client.HttpAsyncClients;
import org.apache.http.util.EntityUtils;
import org.w3c.dom.Document;
import org.xml.sax.SAXException;
public class AsyncWebServiceClient {
private static final String SOAP_ACTION = "http://tempuri.org/HelloWorld";
private static final String ENDPOINT = "http://localhost/HelloWorldService/HelloWorldService.asmx";
private static final String SOAP_REQUEST = "<?xml version=\"1.0\" encoding=\"utf-8\"?>\n"
+ "<soap:Envelope xmlns:xsi=\"http://www.w3.org/2001/XMLSchema-instance\"\n"
+ " xmlns:xsd=\"http://www.w3.org/2001/XMLSchema\"\n"
+ " xmlns:soap=\"http://schemas.xmlsoap.org/soap/envelope/\">\n"
+ " <soap:Body>\n" + " <HelloWorld xmlns=\"http://tempuri.org/\" />\n" + " </soap:Body>\n"
+ "</soap:Envelope>";
public static void main(String[] args) throws Exception {
HttpClient httpClient = HttpAsyncClients.createDefault();
CloseableHttpAsyncClient httpclient = (CloseableHttpAsyncClient) httpClient;
httpclient.start();
try {
HttpPost httpPost = new HttpPost(ENDPOINT);
httpPost.setHeader("Content-Type", "text/xml;charset=UTF-8");
httpPost.setHeader("SOAPAction", SOAP_ACTION);
StringEntity entity = new StringEntity(SOAP_REQUEST, ContentType.TEXT_XML.withCharset(StandardCharsets.UTF_8));
httpPost.setEntity(entity);
Future<HttpResponse> future = httpclient.execute(httpPost, new FutureCallback<HttpResponse>() {
@Override
public void completed(HttpResponse response) {
try {
HttpEntity responseEntity = response.getEntity();
String responseBody = EntityUtils.toString(responseEntity);
System.out.println(responseBody);
Document document = DocumentBuilderFactory.newInstance()
.newDocumentBuilder()
.parse(IOUtils.toInputStream(responseBody, StandardCharsets.UTF_8));
QName qName = new QName("http://tempuri.org/", "HelloWorldResponse");
SOAPBody soapBody = MessageFactory.newInstance().createMessage().getSOAPBody();
Document responseDocument = soapBody.getOwnerDocument();
SOAPHeader soapHeader = responseDocument.createElementNS("http://schemas.xmlsoap.org/soap/envelope/", "soap:Header");
soapBody.addChildElement(qName).appendChild(responseDocument.createTextNode("Hello World!"));
SOAPMessage soapMessage = MessageFactory.newInstance().createMessage(new MimeHeaders(),
IOUtils.toInputStream(responseDocument.getDocumentElement().getTextContent(),
StandardCharsets.UTF_8));
String soapResponse = IOUtils.toString(soapMessage.getSOAPPart().getContent(),
StandardCharsets.UTF_8);
System.out.println(soapResponse);
} catch (IOException | UnsupportedOperationException | SOAPException | ParserConfigurationException | SAXException | TransformerFactoryConfigurationError | TransformerException e) {
e.printStackTrace();
}
}
@Override
public void failed(Exception ex) {
ex.printStackTrace();
}
@Override
public void cancelled() {
}
});
HttpResponse response = future.get();
System.out.println(response);
} finally {
httpclient.close();
}
}
}
```
以上代码使用Apache HttpClient库异步地调用了一个基于SOAP协议的Web Service,实现了将响应报文解析为SOAP消息的功能。在代码中,我们使用了Java自带的XML解析器和SOAP库,也可以使用第三方库实现相同的功能。
相关推荐
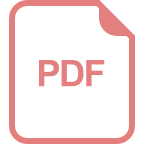
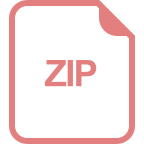
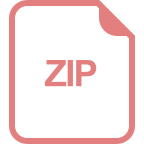












