解释下面的代码所用到的动态规划算法#include <stdio.h> #include <string.h> #define MAX_LEN 100 #define MAX_FREQ 1000000 struct Route { double freq; char word[MAX_LEN]; }; double FREQ[MAX_FREQ]; // 假设FREQ为全局变量,存储词频信息 void calc(char* sentence, int* DAG, int idx, struct Route* route) { int N = strlen(sentence); route[N].freq = 0.0; strcpy(route[N].word, ""); for (idx = N-1; idx >= 0; idx--) { double max_freq = -1.0; int max_idx = -1; for (int x = 0; x < DAG[idx]; x++) { double freq = FREQ[sentence[idx]][x+1]; if (freq > max_freq) { max_freq = freq; max_idx = x; } } route[idx].freq = max_freq + route[max_idx+1].freq; strncpy(route[idx].word, sentence+idx, max_idx+1); route[idx].word[max_idx+1] = '\0'; } } int main() { char sentence[MAX_LEN] = "我爱自然语言处理"; int DAG[MAX_LEN] = {1, 2, 3, 4, 5, 6, 7, 8}; // 假设DAG为全局变量,存储词图信息 struct Route route[MAX_LEN]; // 假设route为全局变量,存储最佳分词路径信息 calc(sentence, DAG, 0, route); // 打印最佳分词路径 for (int i = 0; i < strlen(sentence); i++) { printf("%s / ", route[i].word); } printf("\n"); return 0; }
时间: 2024-04-08 09:31:58 浏览: 68
这段代码使用了动态规划算法来解决最佳分词问题。
首先,定义了一个结构体`Route`用于存储每个位置的最佳分词路径信息。结构体中包含一个词频属性`freq`和一个字符数组`word`用于存储当前位置的最佳分词。
然后,定义了一个全局变量`FREQ[MAX_FREQ]`,用于存储词频信息。
接下来是`calc`函数,它接收一个句子、词图数组`DAG`、当前位置索引和最佳分词路径数组`route`作为参数。在函数内部,首先获取句子的长度,并将最后一个位置的最佳分词路径设置为初始值(频率为0.0,词为空字符串)。
然后,从句子的倒数第二个位置开始遍历到第一个位置,计算每个位置的最佳分词路径。在每个位置上,通过遍历词图数组中的每个节点,获取与当前位置相连的节点的词频,并找到词频最大的节点。这里的动态规划思想体现在,通过比较不同节点的词频大小来选择最佳的分词路径。
然后,将当前位置的最佳分词路径频率设置为当前节点的词频加上下一个节点的最佳分词路径频率,并将当前位置的最佳分词设置为从当前位置到下一个节点之间的字符。
最后,在`main`函数中定义了一个句子数组`sentence`、一个词图数组`DAG`和一个最佳分词路径数组`route`。然后调用`calc`函数对句子进行分词,最后打印出最佳分词路径。
综上所述,这段代码利用动态规划算法计算了给定句子的最佳分词路径,以及每个位置的词频信息。
相关问题
#include <stdio.h> #include <stdlib.h> #include <string.h> #define MAX_NAME_LEN 20 #define MAX_ADDRESS_LEN 50 #define MAX_SEX_LEN 5 #define MAX_PHONE_LEN 11 #define MAX_STUDENT_NUM 1000 // 定义学生结构体 typedef struct { char name[MAX_NAME_LEN]; char address[MAX_ADDRESS_LEN]; char SEX[MAX_SEX_LEN]; char phone[MAX_PHONE_LEN]; } Student; // 定义通讯录结构体 typedef struct { Student students[MAX_STUDENT_NUM]; int num_students; } AddressBook;请写一段修改学生信息的代码
好的,可以参考下面的代码实现修改学生信息:
```c
void modifyStudentInfo(AddressBook *addressBook, int index) {
Student *student = &(addressBook->students[index]);
printf("请输入学生姓名:\n");
scanf("%s", student->name);
printf("请输入学生地址:\n");
scanf("%s", student->address);
printf("请输入学生性别:\n");
scanf("%s", student->SEX);
printf("请输入学生电话号码:\n");
scanf("%s", student->phone);
printf("修改成功!\n");
}
```
这个函数接受两个参数,一个是通讯录结构体指针,另一个是要修改的学生在通讯录中的下标。函数会根据用户输入来修改学生的信息,修改完成后会输出提示信息。注意,函数没有对用户输入进行校验,实际应用中需要加入相应的校验逻辑。
请优化这段代码,执行ioctl报错无效的参数:#include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> #include <sys/socket.h> #include <sys/ioctl.h> #include <netinet/in.h> #include <net/if.h> #include <arpa/inet.h> #include <linux/route.h> int main() { int fd; struct rtentry route; struct sockaddr_in *addr; memset(&route, 0, sizeof(route)); route.rt_dst.sa_family = AF_INET; addr = (struct sockaddr_in *)&route.rt_gateway; addr->sin_family = AF_INET; fd = socket(AF_INET, SOCK_DGRAM, 0); if (ioctl(fd, SIOCRTMSG, &route) == -1) { perror("ioctl"); exit(1); } close(fd); printf("Gateway address: %s\n", inet_ntoa(addr->sin_addr)); return 0; }
这段代码的问题在于使用了已经过时的 SIOCRTMSG ioctl 命令,并且没有对错误进行恰当的处理。可以使用更现代化的方法,如使用 netlink socket 来获取网关地址。以下是更新后的代码:
```c++
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <linux/netlink.h>
#include <linux/rtnetlink.h>
#include <arpa/inet.h>
#define MAX_PAYLOAD 1024
int main() {
int sockfd;
struct sockaddr_nl sa;
struct nlmsghdr *nlh;
char buffer[MAX_PAYLOAD];
struct rtmsg *rtm;
struct rtattr *rta;
int len;
memset(&sa, 0, sizeof(sa));
sa.nl_family = AF_NETLINK;
nlh = (struct nlmsghdr *)buffer;
rtm = (struct rtmsg *)NLMSG_DATA(nlh);
nlh->nlmsg_len = NLMSG_LENGTH(sizeof(struct rtmsg));
nlh->nlmsg_type = RTM_GETROUTE;
nlh->nlmsg_flags = NLM_F_REQUEST | NLM_F_DUMP;
sockfd = socket(AF_NETLINK, SOCK_RAW, NETLINK_ROUTE);
if (sockfd == -1) {
perror("socket");
exit(1);
}
if (bind(sockfd, (struct sockaddr *)&sa, sizeof(sa)) == -1) {
perror("bind");
exit(1);
}
if (send(sockfd, nlh, nlh->nlmsg_len, 0) == -1) {
perror("send");
exit(1);
}
while ((len = recv(sockfd, buffer, MAX_PAYLOAD, 0)) > 0) {
for (nlh = (struct nlmsghdr *)buffer; NLMSG_OK(nlh, len); nlh = NLMSG_NEXT(nlh, len)) {
if (nlh->nlmsg_type == NLMSG_DONE) {
break;
} else if (nlh->nlmsg_type == NLMSG_ERROR) {
perror("recv");
exit(1);
} else if (nlh->nlmsg_type == RTM_NEWROUTE) {
rtm = (struct rtmsg *)NLMSG_DATA(nlh);
if (rtm->rtm_table != RT_TABLE_MAIN) {
continue;
}
rta = (struct rtattr *)RTM_RTA(rtm);
len = RTM_PAYLOAD(nlh);
for (; RTA_OK(rta, len); rta = RTA_NEXT(rta, len)) {
if (rta->rta_type == RTA_GATEWAY) {
struct in_addr *addr = (struct in_addr *)RTA_DATA(rta);
printf("Gateway address: %s\n", inet_ntoa(*addr));
close(sockfd);
return 0;
}
}
}
}
}
close(sockfd);
return 0;
}
```
这个程序使用 netlink socket 发送 RTM_GETROUTE 请求来获取路由表,并遍历路由表中的每个条目,找到目标路由条目,并从中提取出网关地址。此方法可以避免使用已经过时的 ioctl 命令,并且可以正确地处理错误。
阅读全文
相关推荐
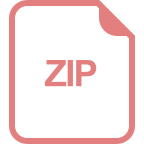
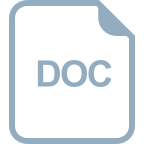










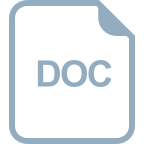