写一段上面代码的C++版本
时间: 2024-02-27 10:58:36 浏览: 90
好的,以下是上面 Python 代码的 C++ 版本:
```c++
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
// 读入图像,转换为灰度图像,并进行二值化处理
Mat img = imread("example.png");
Mat gray, thresh;
cvtColor(img, gray, COLOR_BGR2GRAY);
threshold(gray, thresh, 127, 255, THRESH_BINARY);
// 找到图像中所有轮廓,并绘制出来
std::vector<std::vector<Point>> contours;
std::vector<Vec4i> hierarchy;
findContours(thresh, contours, hierarchy, RETR_TREE, CHAIN_APPROX_SIMPLE);
drawContours(img, contours, -1, Scalar(0,255,0), 2);
// 对于每个轮廓,计算面积和颜色平均值,并确定色相范围
for (int i = 0; i < contours.size(); i++) {
double area = contourArea(contours[i]);
Scalar mean_val = mean(img, thresh);
Mat hsv;
cvtColor(Mat(1, 1, CV_8UC3, mean_val), hsv, COLOR_BGR2HSV);
int h = hsv.at<Vec3b>(0,0)[0];
int lower_h = h - 10;
int upper_h = h + 10;
Scalar lower = Scalar(lower_h, 50, 50);
Scalar upper = Scalar(upper_h, 255, 255);
Mat mask;
inRange(cvtColor(img, mask, COLOR_BGR2HSV), lower, upper, mask);
int color_pixels = countNonZero(mask);
// 计算颜色百分比并输出
double color_percent = (color_pixels / area) * 100;
std::cout << "Contour area: " << area << std::endl;
std::cout << "Color mean: " << mean_val << std::endl;
std::cout << "Color percent: " << color_percent << std::endl;
}
imshow("image", img);
waitKey(0);
destroyAllWindows();
return 0;
}
```
请注意,由于 OpenCV 的 C++ 接口与 Python 接口有所不同,因此代码结构和语法可能略有不同,但算法流程与 Python 版本基本相同。
阅读全文
相关推荐
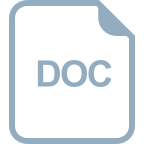
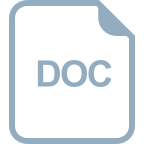
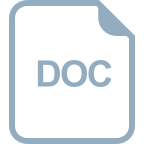















